fastapi写一个后台管理系统
时间: 2023-11-11 22:05:48 浏览: 182
好的,我可以为您介绍如何使用FastAPI来编写后台管理系统。FastAPI是一个高性能的Web框架,它支持异步请求和响应,提供了快速的API开发工具。您可以使用FastAPI来构建可扩展的Web应用程序,它提供了内置的数据验证,自动生成文档和基于OpenAPI的API交互式测试等功能。
为了构建后台管理系统,您可以使用FastAPI的路由和请求处理器来处理用户请求和响应。此外,您还可以使用FastAPI的ORM和数据库连接工具来连接数据库并处理数据。
以下是一个基本示例,可以用来演示如何使用FastAPI构建后台管理系统:
```python
from fastapi import FastAPI, HTTPException
from pydantic import BaseModel
from typing import List
app = FastAPI()
# 数据库用户查找信息的实体对象
class User(BaseModel):
id: int
name: str
email: str
# 存储用户信息的列表
fake_user_db = [
{"id": 1, "name": "John Doe", "email": "johndoe@example.com"},
{"id": 2, "name": "Alice Smith", "email": "alicesmith@example.com"},
{"id": 3, "name": "Bob Brown", "email": "bobbrown@example.com"},
]
# 获取所有用户的列表
@app.get("/users/", response_model=List[User])
async def read_users(skip: int = 0, limit: int = 100):
return fake_user_db[skip : skip + limit]
# 获取某个用户的详细信息
@app.get("/users/{user_id}", response_model=User)
async def read_user(user_id: int):
user = next((u for u in fake_user_db if u["id"] == user_id), None)
if user is None:
raise HTTPException(status_code=404, detail="User not found")
return user
# 创建新用户的方法
@app.post("/users/", response_model=User)
async def create_user(user: User):
fake_user_db.append(user.dict())
return user
# 更新某个用户的信息
@app.put("/users/{user_id}", response_model=User)
async def update_user(user_id: int, user: User):
current_user = next((u for u in fake_user_db if u["id"] == user_id), None)
if current_user is None:
raise HTTPException(status_code=404, detail="User not found")
current_user.update(user.dict())
return user
```
这个例子中,我们创建了一个名为`User`的模型来表示用户实体。我们还定义了一些路由和请求处理器来展示如何创建、从数据库中读取和更新用户。您可以根据您的特定业务需求来扩展这个示例,以满足您的具体要求。
阅读全文
相关推荐
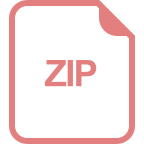




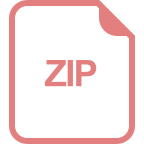
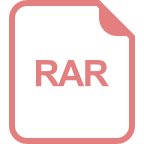
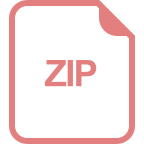
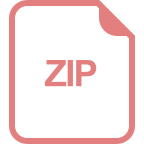
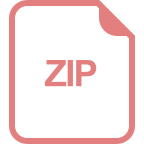
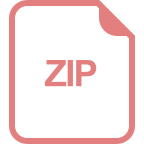
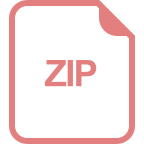
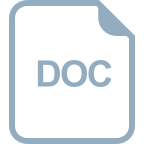
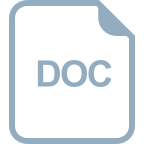


