canvas path中R如何使用
时间: 2024-06-09 18:05:30 浏览: 120
在Canvas中,R代表曲线的控制点,它用于创建二次或三次贝塞尔曲线。在二次贝塞尔曲线中,R表示曲线的控制点,它是一个二元组,它指定了曲线的弯曲方向和程度。在三次贝塞尔曲线中,R表示曲线的第一个控制点,它是一个二元组,它指定了曲线的起始方向和程度。
要在Canvas中使用R,您可以使用以下方法创建二次贝塞尔曲线:
```
context.quadraticCurveTo(Rx, Ry, x, y);
```
其中,Rx和Ry是控制点的坐标,x和y是曲线的终点坐标。
要创建三次贝塞尔曲线,您可以使用以下方法:
```
context.bezierCurveTo(R1x, R1y, R2x, R2y, x, y);
```
其中,R1x和R1y是第一个控制点的坐标,R2x和R2y是第二个控制点的坐标,x和y是曲线的终点坐标。
请注意,使用R创建曲线需要一些绘图基础知识。如果您不熟悉曲线绘制,建议您先学习一些基本知识。
相关问题
如何使用canvas path中的r
在Canvas中,r通常指的是一个圆的半径。在绘制圆形路径时,可以使用`arc()`方法。这个方法需要传入的参数包括圆心的x、y坐标、半径r、起始角度和结束角度,以及一个可选的布尔值参数,用于指定绘制的方向。
例如,下面的代码将在Canvas中绘制一个半径为50像素的圆:
```javascript
const canvas = document.getElementById('myCanvas');
const ctx = canvas.getContext('2d');
ctx.beginPath();
ctx.arc(100, 100, 50, 0, 2 * Math.PI);
ctx.stroke();
```
在这个例子中,`arc()`方法中的第一个参数指定了圆心的x坐标,第二个参数指定了圆心的y坐标,第三个参数指定了圆的半径,第四个参数指定了起始角度,这里是0,表示从圆的最右侧开始绘制。第五个参数指定了结束角度,这里是2 * Math.PI,表示绘制整个圆。最后,`stroke()`方法用于绘制路径。
如何使用webgl在dicom文件上使用canvas来画出影像左右(L,R)显示具体代码
以下是一个简单的WebGL和Canvas代码示例,用于在DICOM文件上绘制影像左右(L,R)显示。请注意,这只是一个基础示例,实际应用中可能需要更多的代码和调整。
```html
<!DOCTYPE html>
<html>
<head>
<title>DICOM WebGL Canvas Demo</title>
<style>
canvas {
width: 100%;
height: 100%;
}
</style>
</head>
<body>
<canvas id="canvas"></canvas>
<script src="https://cdnjs.cloudflare.com/ajax/libs/three.js/r128/three.min.js"></script>
<script src="https://unpkg.com/cornerstone-core/dist/cornerstone.js"></script>
<script src="https://unpkg.com/cornerstone-webgl/dist/cornerstoneWebgl.js"></script>
<script src="https://unpkg.com/cornerstone-math/dist/cornerstoneMath.js"></script>
<script>
// Load DICOM file
cornerstoneWADOImageLoader.external.cornerstone = cornerstone;
const imageId = 'wadouri:/path/to/dicom/file';
cornerstone.loadImage(imageId).then(function (image) {
// Setup WebGL context
const canvas = document.getElementById('canvas');
const renderer = new THREE.WebGLRenderer({ canvas });
const scene = new THREE.Scene();
const camera = new THREE.PerspectiveCamera(45, canvas.width / canvas.height, 0.1, 10000);
camera.position.z = 300;
// Setup cube
const cubeGeometry = new THREE.BoxGeometry(256, 256, 256);
const cubeMaterial = new THREE.MeshBasicMaterial({
map: new THREE.CanvasTexture(canvas)
});
const cube = new THREE.Mesh(cubeGeometry, cubeMaterial);
scene.add(cube);
// Setup texture
const texture = cornerstoneWebGLTextureCache.getTexture(imageId);
const textureWidth = THREE.Math.nearestPowerOfTwo(texture.width);
const textureHeight = THREE.Math.nearestPowerOfTwo(texture.height);
const canvasTexture = new THREE.CanvasTexture(canvas);
canvasTexture.wrapS = THREE.ClampToEdgeWrapping;
canvasTexture.wrapT = THREE.ClampToEdgeWrapping;
// Setup L/R views
const viewLeft = cornerstoneMath.matrix.new3x3();
const viewRight = cornerstoneMath.matrix.new3x3();
const viewUp = cornerstoneMath.matrix.new3x3();
const viewDown = cornerstoneMath.matrix.new3x3();
const viewRotation = cornerstoneMath.matrix.new4x4();
const viewTranslation = cornerstoneMath.vector3.create();
const viewAxis = cornerstoneMath.vector3.create();
const viewUpVector = cornerstoneMath.vector3.create();
const right = cornerstoneMath.vector3.create(1, 0, 0);
const up = cornerstoneMath.vector3.create(0, 1, 0);
const axis = cornerstoneMath.vector3.create();
const angle = Math.PI / 2;
cornerstoneTools.orientation.getOrientationString(image.imageId);
if (image.rowCosines[0] < 0) {
cornerstoneMath.vector3.negate(right, right);
}
if (image.columnCosines[1] > 0) {
cornerstoneMath.vector3.negate(up, up);
}
cornerstoneMath.vector3.cross(up, right, axis);
cornerstoneMath.matrix.setFromAxisAngle(axis, angle, viewLeft);
cornerstoneMath.matrix.setFromAxisAngle(axis, -angle, viewRight);
cornerstoneMath.vector3.cross(right, axis, viewUpVector);
cornerstoneMath.matrix.setFromAxisAngle(viewUpVector, angle, viewUp);
cornerstoneMath.matrix.setFromAxisAngle(viewUpVector, -angle, viewDown);
// Render loop
function animate() {
requestAnimationFrame(animate);
// Rotate cube
cube.rotation.y += 0.01;
// Update L/R views
cornerstoneMath.matrix.multiply(viewUp, viewLeft, viewRotation);
cornerstoneMath.vector3.scale(right, -0.5 * textureWidth, viewTranslation);
cornerstoneMath.vector3.add(viewTranslation, cornerstoneMath.vector3.scale(up, -0.5 * textureHeight));
cornerstoneMath.matrix.setTranslation(viewRotation, viewTranslation);
canvasTexture.needsUpdate = true;
renderer.render(scene, camera);
cornerstoneMath.matrix.multiply(viewUp, viewRight, viewRotation);
cornerstoneMath.vector3.scale(right, 0.5 * textureWidth, viewTranslation);
cornerstoneMath.vector3.add(viewTranslation, cornerstoneMath.vector3.scale(up, -0.5 * textureHeight));
cornerstoneMath.matrix.setTranslation(viewRotation, viewTranslation);
canvasTexture.needsUpdate = true;
renderer.render(scene, camera);
}
animate();
});
</script>
</body>
</html>
```
请注意,这只是一个基础示例,实际应用中可能需要更多的代码和调整。
阅读全文
相关推荐
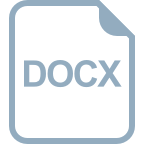
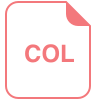
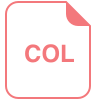


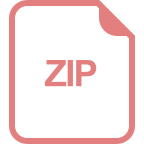
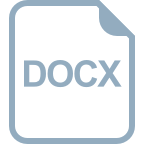
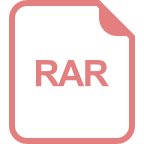
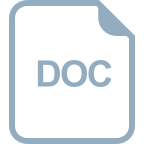
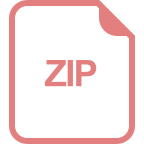


