建立一个描述学生成绩的无序链表,各结点内容如表8.3所示。计算各学生的总成绩,并输出链表中各学生结点的信息内容。最后删除链表,收回链表占用的存储空间。建立无序链表、计算总成绩、输出链表、删除链表各用一个函数实现。在主函数中调用四个函数完成上述操作。
时间: 2024-03-13 13:16:36 浏览: 44
好的,这是一个关于链表的操作题目。下面是对应的代码实现:
```c++
#include <iostream>
using namespace std;
// 学生成绩结点结构体
struct Node {
string name; // 学生姓名
int score1; // 学生成绩1
int score2; // 学生成绩2
int score3; // 学生成绩3
int total; // 学生总成绩
Node* next; // 下一个结点指针
};
// 建立无序链表
Node* createList() {
Node* head = new Node;
head->next = nullptr;
Node* p = head;
int n;
cout << "请输入学生数量:";
cin >> n;
for (int i = 0; i < n; i++) {
Node* node = new Node;
cout << "请输入第" << i + 1 << "个学生的姓名和三门成绩:";
cin >> node->name >> node->score1 >> node->score2 >> node->score3;
node->total = node->score1 + node->score2 + node->score3;
p->next = node;
p = p->next;
}
return head;
}
// 计算总成绩
void calculateTotal(Node* head) {
Node* p = head->next;
while (p) {
p->total = p->score1 + p->score2 + p->score3;
p = p->next;
}
}
// 输出链表
void printList(Node* head) {
Node* p = head->next;
while (p) {
cout << "姓名:" << p->name << ",成绩1:" << p->score1
<< ",成绩2:" << p->score2 << ",成绩3:" << p->score3
<< ",总成绩:" << p->total << endl;
p = p->next;
}
}
// 删除链表
void deleteList(Node* head) {
Node* p = head;
while (p) {
Node* q = p;
p = p->next;
delete q;
}
}
int main() {
Node* head = createList(); // 建立链表
calculateTotal(head); // 计算总成绩
printList(head); // 输出链表
deleteList(head); // 删除链表
return 0;
}
```
你可以尝试运行一下这个程序,并输入学生信息,查看结果是否符合题目要求。
阅读全文
相关推荐
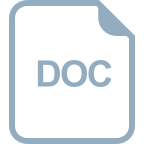
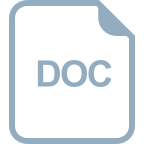
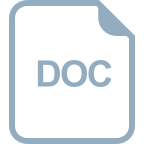
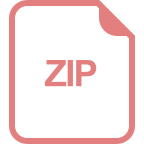
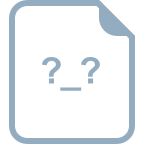
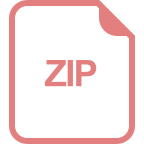
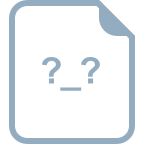
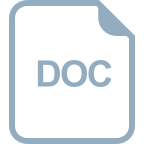
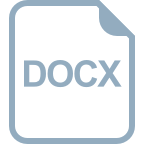
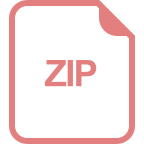
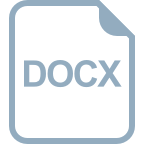
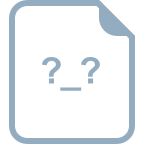
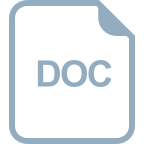
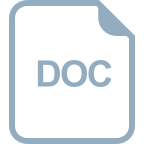
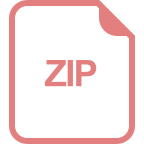