windows下c++多线程
时间: 2023-10-13 15:07:51 浏览: 52
在Windows系统下,使用C语言实现多线程可以使用Windows API提供的线程函数。
Windows API提供了以下线程函数:
1. CreateThread():创建一个新的线程。
2. GetCurrentThread():获取当前线程的句柄。
3. GetCurrentThreadId():获取当前线程的ID。
4. ExitThread():终止当前线程。
5. Sleep():将当前线程挂起一段时间。
6. WaitForSingleObject():等待一个对象的信号。
7. WaitForMultipleObjects():等待多个对象的信号。
下面是一个简单的例子,展示如何使用Windows API创建多线程:
```c
#include <windows.h>
#include <stdio.h>
DWORD WINAPI threadFunc(LPVOID lpParam) {
printf("Thread %d is running...\n", GetCurrentThreadId());
return 0;
}
int main() {
HANDLE hThread;
DWORD threadId;
hThread = CreateThread(NULL, 0, threadFunc, NULL, 0, &threadId);
if (hThread == NULL) {
printf("Failed to create thread.\n");
return 1;
}
printf("Main thread is waiting for thread %d to complete...\n", threadId);
WaitForSingleObject(hThread, INFINITE);
CloseHandle(hThread);
printf("All threads have completed.\n");
return 0;
}
```
在上面的例子中,使用CreateThread()函数创建了一个新线程,并使用WaitForSingleObject()函数等待新线程执行完毕。注意,使用完线程句柄后需要使用CloseHandle()函数释放资源。
相关推荐
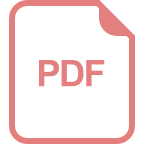
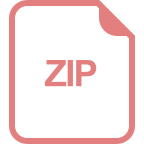














