Unity3D Shader图像扭曲过场效果
时间: 2024-01-20 20:02:46 浏览: 168
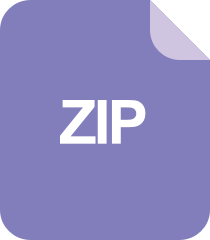
图像扭曲特效
要实现图像扭曲过场效果,可以使用Unity3D中的Shader。具体实现步骤如下:
1. 创建一个新的Shader,并将其属性设置为Unlit/Texture。
2. 在Shader中添加一个名为“Distortion”的属性,类型为2D纹理,用于存储扭曲图像。
3. 在Shader中添加一个名为“DistortionStrength”的属性,类型为Range,用于控制扭曲强度。
4. 在Shader的片段着色器中,使用tex2D函数获取原始纹理的颜色,并使用uv坐标对扭曲图像进行采样。
5. 将扭曲图像的采样值与“DistortionStrength”属性相乘,并将结果添加到原始颜色中。
6. 最后,将新的颜色值输出到屏幕。
下面是一个简单的Shader示例:
```
Shader "Custom/DistortionTransition" {
Properties {
_MainTex ("Texture", 2D) = "white" {}
_Distortion ("Distortion", 2D) = "white" {}
_DistortionStrength ("Distortion Strength", Range(0.0, 1.0)) = 0.5
}
SubShader {
Pass {
CGPROGRAM
#pragma vertex vert
#pragma fragment frag
#include "UnityCG.cginc"
struct appdata {
float4 vertex : POSITION;
float2 uv : TEXCOORD0;
};
struct v2f {
float2 uv : TEXCOORD0;
float4 vertex : SV_POSITION;
};
sampler2D _MainTex;
sampler2D _Distortion;
float _DistortionStrength;
v2f vert (appdata v) {
v2f o;
o.vertex = UnityObjectToClipPos(v.vertex);
o.uv = v.uv;
return o;
}
fixed4 frag (v2f i) : SV_Target {
// Get the original color
fixed4 texColor = tex2D(_MainTex, i.uv);
// Get the distortion value
float4 distortion = tex2D(_Distortion, i.uv);
// Calculate the distortion offset
float2 offset = (distortion.rg * 2.0 - 1.0) * _DistortionStrength;
// Apply the distortion to the UV coordinates
float2 distortedUV = i.uv + offset;
// Get the color from the distorted UV coordinates
fixed4 distortedColor = tex2D(_MainTex, distortedUV);
// Add the distortion to the original color
return texColor + (distortedColor - texColor);
}
ENDCG
}
}
FallBack "Diffuse"
}
```
在使用这个Shader时,可以将原始纹理作为“_MainTex”属性的值,将扭曲图像作为“_Distortion”属性的值,然后通过修改“_DistortionStrength”属性的值来控制扭曲强度。
希望这个示例能够帮助你实现所需的效果。
阅读全文
相关推荐
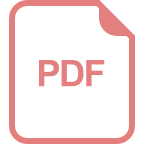
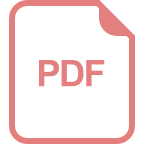
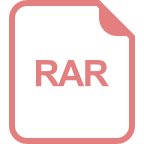
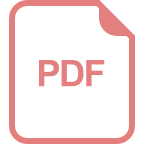
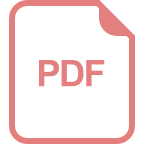
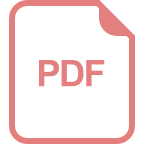
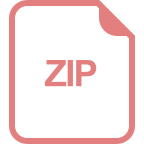
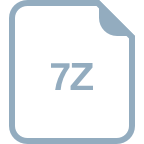
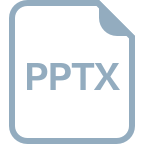
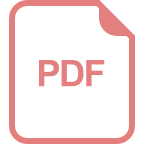
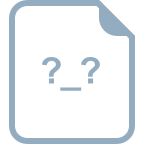
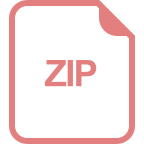
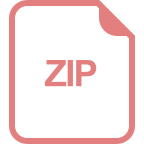