java api操作ES
时间: 2023-10-23 13:21:22 浏览: 96
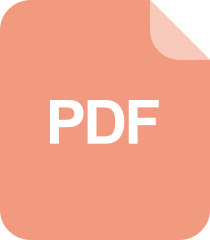
ES学习之 javaAPI实操演示
Java API可以用于操作Elasticsearch(ES)搜索引擎,以下是使用Java API进行ES操作的基本步骤:
1. 导入依赖
在项目中添加Elasticsearch Java API的依赖,例如:
```xml
<dependency>
<groupId>org.elasticsearch.client</groupId>
<artifactId>elasticsearch-rest-high-level-client</artifactId>
<version>7.14.1</version>
</dependency>
```
2. 创建连接
使用Java API连接ES集群,例如:
```java
RestHighLevelClient client = new RestHighLevelClient(
RestClient.builder(new HttpHost("localhost", 9200, "http")));
```
3. 创建索引
使用Java API创建索引,例如:
```java
CreateIndexRequest request = new CreateIndexRequest("my_index");
client.indices().create(request, RequestOptions.DEFAULT);
```
4. 添加文档
使用Java API向索引添加文档,例如:
```java
IndexRequest request = new IndexRequest("my_index");
request.id("1");
String jsonString = "{" +
"\"name\":\"John\"," +
"\"age\":30," +
"\"city\":\"New York\"" +
"}";
request.source(jsonString, XContentType.JSON);
client.index(request, RequestOptions.DEFAULT);
```
5. 搜索文档
使用Java API搜索文档,例如:
```java
SearchRequest request = new SearchRequest("my_index");
SearchSourceBuilder builder = new SearchSourceBuilder();
builder.query(QueryBuilders.matchQuery("name", "John"));
request.source(builder);
SearchResponse response = client.search(request, RequestOptions.DEFAULT);
```
6. 关闭连接
使用完Java API后,需要关闭连接,例如:
```java
client.close();
```
以上就是使用Java API操作ES的基本步骤。具体的操作方式和方法需要根据具体的需求来进行选择。同时,需要注意ES版本和Java API版本的兼容性。
阅读全文
相关推荐
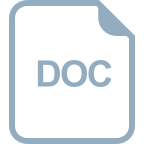
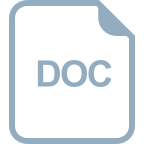
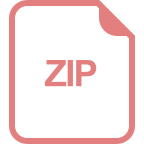
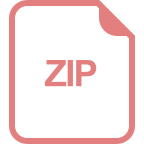

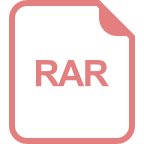
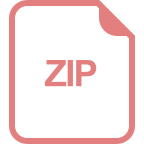
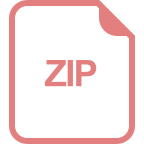
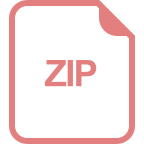
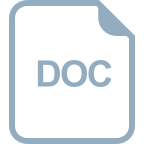






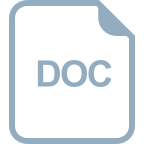