opencv 读取大量图片
时间: 2023-11-09 22:08:46 浏览: 70
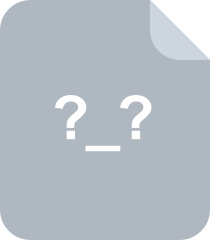
opencv 读取图像
在opencv中,可以使用cv::imread函数读取单张图像,但是如果需要读取大量图片,可以使用以下方法:
1. 使用循环读取单张图片
可以使用循环读取每张图片,然后将它们存储在vector中。示例代码如下:
```
#include <opencv2/opencv.hpp>
#include <iostream>
#include <vector>
using namespace cv;
using namespace std;
int main(int argc, char** argv)
{
vector<Mat> images;
for(int i=1; i<=100; i++)
{
string filename = "image" + to_string(i) + ".jpg";
Mat image = imread(filename);
if(image.empty())
{
cout << "Could not read image " << filename << endl;
break;
}
images.push_back(image);
}
cout << "Number of images read: " << images.size() << endl;
return 0;
}
```
2. 使用glob函数读取多张图片
可以使用glob函数读取所有符合某个模式的图片。示例代码如下:
```
#include <opencv2/opencv.hpp>
#include <iostream>
#include <vector>
using namespace cv;
using namespace std;
int main(int argc, char** argv)
{
vector<Mat> images;
string pattern = "path/to/images/*.jpg"; // 指定匹配模式
vector<String> filenames;
glob(pattern, filenames);
for(int i=0; i<filenames.size(); i++)
{
Mat image = imread(filenames[i]);
if(image.empty())
{
cout << "Could not read image " << filenames[i] << endl;
break;
}
images.push_back(image);
}
cout << "Number of images read: " << images.size() << endl;
return 0;
}
```
3. 使用OpenCV的数据集读取器
OpenCV中提供了数据集读取器,可以用来读取大量的图片数据集,例如MNIST、CIFAR等。示例代码如下:
```
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main(int argc, char** argv)
{
string dataset_path = "path/to/dataset"; // 指定数据集路径
Ptr<ml::TrainData> dataset = ml::TrainData::loadFromCSV(dataset_path,
0, // 标签列
-1, // 最后一列为特征列
-1, // 不使用行索引
"ord[0,1,2,3]"); // 类别标签为0,1,2,3
cout << "Number of images read: " << dataset->getNSamples() << endl;
return 0;
}
```
以上三种方法可以根据实际需求选择使用。
阅读全文
相关推荐

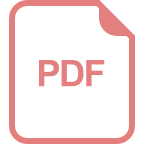
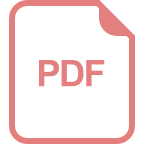
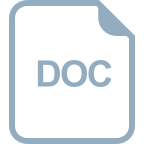
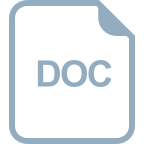


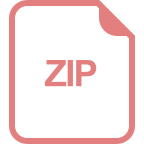
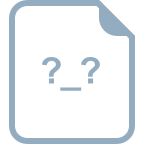
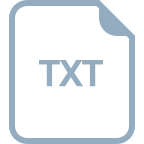
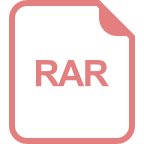
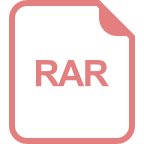
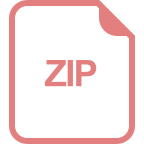
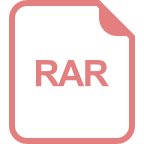
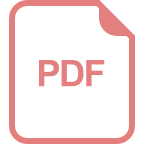