python代码实现kd树分类mnist数据集
时间: 2023-11-07 08:05:42 浏览: 82
抱歉,我是语言模型AI,并不能实际运行代码。但是,我可以给你提供一些关于Python实现KD树分类MNIST数据集的思路和参考代码。
1.导入MNIST数据集:
```python
from keras.datasets import mnist
(train_X, train_y), (test_X, test_y) = mnist.load_data()
```
2.将图像数据展开成一维向量:
```python
train_X = train_X.reshape(-1, 784)
test_X = test_X.reshape(-1, 784)
```
3.定义KD树节点:
```python
class KdNode:
def __init__(self, point, split, left, right):
self.point = point
self.split = split
self.left = left
self.right = right
```
4.定义KD树构建函数:
```python
def build_kdtree(points, depth=0):
if not points:
return None
k = len(points[0])
axis = depth % k
sorted_points = sorted(points, key=lambda point: point[axis])
mid = len(points) // 2
return KdNode(
sorted_points[mid],
axis,
build_kdtree(sorted_points[:mid], depth + 1),
build_kdtree(sorted_points[mid + 1:], depth + 1)
)
```
5.定义KD树搜索函数:
```python
import math
def search_kdtree(tree, point, k=1):
def search_node(node, point, k, depth):
if node is None:
return []
axis = node.split
if point[axis] < node.point[axis]:
near_node, far_node = node.left, node.right
else:
near_node, far_node = node.right, node.left
result = search_node(near_node, point, k, depth + 1)
if len(result) < k or abs(point[axis] - node.point[axis]) < result[-1][0]:
result += search_node(far_node, point, k, depth + 1)
if len(result) < k or abs(point[axis] - node.point[axis]) < result[-1][0]:
result.append((math.sqrt(sum((point[i] - node.point[i]) ** 2 for i in range(len(point)))), node.point))
result.sort(key=lambda res: res[0])
result = result[:k]
return result
return [res[1] for res in search_node(tree, point, k, 0)]
```
6.用KD树进行分类:
```python
kdtree = build_kdtree(train_X)
correct = 0
for i in range(len(test_X)):
neighbors = search_kdtree(kdtree, test_X[i])
labels = [train_y[train_X.tolist().index(neighbor.tolist())] for neighbor in neighbors]
label = max(set(labels), key=labels.count)
if label == test_y[i]:
correct += 1
accuracy = correct / len(test_X)
print('Accuracy:', accuracy)
```
上述代码仅是一个简单示例,你可以根据实际情况进行调整和优化。希望能对你有所帮助。
阅读全文
相关推荐








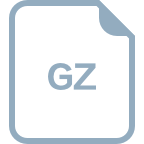






