用qml写一个天气预报
时间: 2023-10-06 16:06:34 浏览: 136
好的,以下是一个简单的天气预报应用程序的 QML 代码示例:
```qml
import QtQuick 2.0
import QtQuick.Controls 2.0
import QtQuick.Layouts 1.3
import QtQuick.Controls.Material 2.0
import QtLocation 5.6
ApplicationWindow {
id: appWindow
visible: true
width: 400
height: 600
// 定义一个变量来存储城市名称
property string city: "北京"
// 定义一个函数来获取天气数据
function getWeatherData(city) {
var xhr = new XMLHttpRequest();
xhr.open("GET", "http://wthrcdn.etouch.cn/weather_mini?city=" + city, true);
xhr.onreadystatechange = function() {
if (xhr.readyState === XMLHttpRequest.DONE && xhr.status === 200) {
var response = JSON.parse(xhr.responseText);
if (response.status === 1000) {
// 更新天气数据
weatherModel.clear();
var forecast = response.data.forecast;
for (var i = 0; i < forecast.length; i++) {
weatherModel.append({
date: forecast[i].date,
high: forecast[i].high,
low: forecast[i].low,
type: forecast[i].type
});
}
} else {
console.log("获取天气数据失败:" + response.desc);
}
}
}
xhr.send();
}
// 定义一个模型来存储天气数据
ListModel {
id: weatherModel
}
// 垂直布局
ColumnLayout {
anchors.fill: parent
spacing: 10
// 文本输入框用于输入城市名称
TextField {
id: cityInput
placeholderText: "输入城市名称"
onAccepted: {
// 获取新城市的天气数据
city = cityInput.text;
getWeatherData(city);
}
}
// 点击按钮获取当前城市的天气数据
Button {
text: "获取天气"
onClicked: {
getWeatherData(city);
}
}
// 天气数据列表
ListView {
id: weatherList
model: weatherModel
delegate: Item {
width: parent.width
height: 70
ColumnLayout {
anchors.fill: parent
spacing: 5
Text {
text: model.date
font.bold: true
}
Text {
text: model.type
}
Text {
text: model.high + "/" + model.low
}
}
}
}
}
// 初始化获取当前城市的天气数据
Component.onCompleted: {
getWeatherData(city);
}
}
```
代码分为以下几部分:
1. 定义一个 `city` 变量来存储城市名称。
2. 定义一个 `getWeatherData(city)` 函数用于获取天气数据。这里使用了 `XMLHttpRequest` 对象来发送 HTTP 请求,并在获取到响应后解析 JSON 数据。
3. 定义一个 `weatherModel` 模型来存储天气数据。
4. 使用 `ColumnLayout` 垂直布局来排列应用程序中的 UI 元素。
5. 在布局中添加一个 `TextField` 用于输入城市名称,一个 `Button` 用于获取天气数据,以及一个 `ListView` 用于显示天气数据。
6. 在 `ListView` 的 `delegate` 中定义一个 `Item` 来显示每一个天气数据。
7. 在 `Component.onCompleted` 中,调用 `getWeatherData(city)` 函数来获取当前城市的天气数据。
这是一个基本的天气预报应用程序,可以根据自己的需要进行扩展和修改。
阅读全文
相关推荐
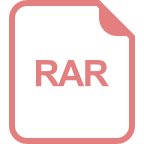
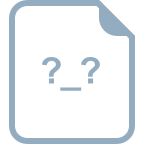
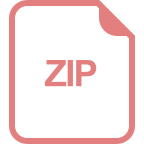
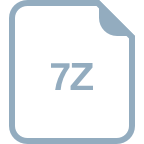
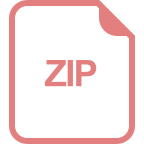
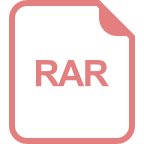
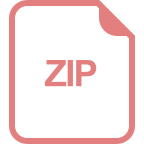
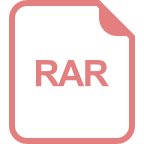
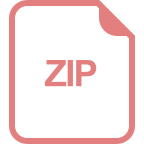
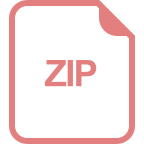
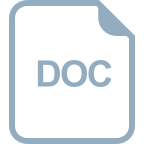
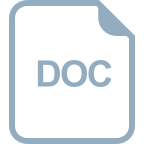


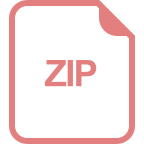
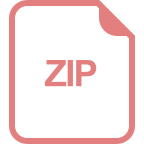