spring_使用Spring编写和使用SOAP Web服务
时间: 2024-06-08 19:08:36 浏览: 122
Spring框架提供了许多方式来编写和使用SOAP Web服务。下面是一些基本的步骤:
1. 导入Spring Web Services库
首先,需要在项目中导入Spring Web Services库。可以使用Maven或Gradle等构建工具添加以下依赖项:
```
<dependency>
<groupId>org.springframework.ws</groupId>
<artifactId>spring-ws-core</artifactId>
<version>3.0.7.RELEASE</version>
</dependency>
```
2. 创建WSDL
Web服务描述语言(WSDL)是一种XML格式,用于描述Web服务的接口、数据类型和协议。可以使用Spring Web Services自动生成WSDL文件。
在Spring中,可以使用`@Endpoint`注解创建一个端点类,并使用`@PayloadRoot`注解指定请求的XML命名空间和元素名称。例如:
```
@Endpoint
public class UserEndpoint {
private static final String NAMESPACE_URI = "http://example.com/users";
@PayloadRoot(namespace = NAMESPACE_URI, localPart = "getUserRequest")
@ResponsePayload
public GetUserResponse getUser(@RequestPayload GetUserRequest request) {
// 处理请求并返回响应
}
}
```
3. 配置Spring Web Services
可以使用Spring配置文件来配置Spring Web Services。以下是一个简单的配置文件示例:
```
<bean id="marshaller" class="org.springframework.oxm.jaxb.Jaxb2Marshaller">
<property name="contextPath" value="com.example.users"/>
</bean>
<bean id="messageFactory" class="org.springframework.ws.soap.saaj.SaajSoapMessageFactory"/>
<bean id="userEndpoint" class="com.example.users.UserEndpoint"/>
<bean class="org.springframework.ws.transport.http.WebServiceExporter">
<property name="messageFactory" ref="messageFactory"/>
<property name="port" value="8080"/>
<property name="serviceMappings">
<props>
<prop key="http://example.com/users">userEndpoint</prop>
</props>
</property>
<property name="defaultEndpoint" ref="userEndpoint"/>
</bean>
```
在这个配置文件中,我们定义了一个JAXB Marshaller用于将Java对象转换为XML,一个SOAP消息工厂用于创建SOAP消息,以及一个Web服务导出器用于将Web服务公开在HTTP端口8080上。
4. 测试Web服务
现在,可以使用SOAP客户端来测试Web服务。可以使用许多不同的SOAP客户端工具,例如SOAPUI或Apache CXF。以下是一个使用Apache CXF的简单Java客户端示例:
```
JaxWsProxyFactoryBean factory = new JaxWsProxyFactoryBean();
factory.setServiceClass(UserService.class);
factory.setAddress("http://localhost:8080/users");
UserService client = (UserService) factory.create();
GetUserRequest request = new GetUserRequest();
request.setId(1);
GetUserResponse response = client.getUser(request);
```
在这个示例中,我们创建了一个JAX-WS代理工厂,并使用它创建了一个Web服务客户端。然后,我们创建一个请求对象并调用Web服务方法。
这就是使用Spring编写和使用SOAP Web服务的基本步骤。当然,这只是一个简单的示例,实际应用中可能需要更复杂的配置和处理。
阅读全文
相关推荐
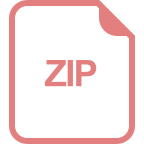
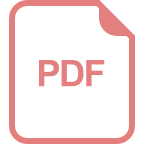
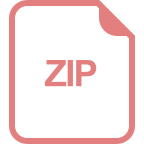














