帮我写一段遍历所有仓库执行打tag操作的C代码
时间: 2024-06-08 08:11:03 浏览: 137
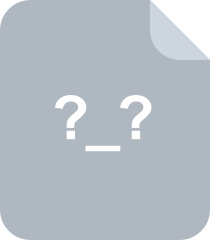
基本C标签遍历数据
假设你使用的是GitHub API,以下是一个遍历所有仓库并执行打标签操作的C代码示例:
```c
#include <stdio.h>
#include <curl/curl.h>
#include <jansson.h>
#define TOKEN "YOUR_GITHUB_TOKEN"
size_t write_callback(char *ptr, size_t size, size_t nmemb, void *userdata) {
// This function is called by libcurl when data is received
// We don't need to do anything with the data but we need to define this function
return size * nmemb;
}
void create_tag(char* owner, char* repo, char* sha, char* tag_name) {
CURL *curl;
CURLcode res;
char url[256];
char auth_header[256];
json_t *json_data;
json_error_t json_error;
// Set up the URL for creating a tag
sprintf(url, "https://api.github.com/repos/%s/%s/git/tags", owner, repo);
// Set up the authentication header
sprintf(auth_header, "Authorization: token %s", TOKEN);
// Create a JSON object that contains the tag data
json_data = json_pack("{s:s, s:s}", "object", sha, "tag", tag_name);
// Initialize libcurl
curl = curl_easy_init();
if (curl) {
// Set the URL
curl_easy_setopt(curl, CURLOPT_URL, url);
// Set the authentication header
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, auth_header);
headers = curl_slist_append(headers, "Content-Type: application/json");
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, headers);
// Set the JSON data to be sent in the request body
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, json_dumps(json_data, JSON_COMPACT));
// Set the write callback function to handle response data
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, write_callback);
// Send the request
res = curl_easy_perform(curl);
// Clean up
curl_easy_cleanup(curl);
curl_slist_free_all(headers);
json_decref(json_data);
}
}
void tag_all_repos() {
CURL *curl;
CURLcode res;
char url[256];
char auth_header[256];
json_t *json_data;
json_t *json_array;
json_t *item;
json_error_t json_error;
// Set up the URL for getting the list of repositories
sprintf(url, "https://api.github.com/user/repos");
// Set up the authentication header
sprintf(auth_header, "Authorization: token %s", TOKEN);
// Initialize libcurl
curl = curl_easy_init();
if (curl) {
// Set the URL
curl_easy_setopt(curl, CURLOPT_URL, url);
// Set the authentication header
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, auth_header);
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, headers);
// Set the write callback function to handle response data
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, write_callback);
// Send the request
res = curl_easy_perform(curl);
// Parse the response data as JSON
json_data = json_loads(curl_easy_strerror(res), 0, &json_error);
// Get the array of repositories from the JSON data
json_array = json_object_get(json_data, "repositories");
// Loop through each repository
for (int i = 0; i < json_array_size(json_array); i++) {
// Get the owner, repo name, and latest commit SHA for the repository
item = json_array_get(json_array, i);
char* owner = json_string_value(json_object_get(item, "owner"));
char* repo_name = json_string_value(json_object_get(item, "name"));
// TODO: Get the latest commit SHA for the repository
// Create a tag for the latest commit
create_tag(owner, repo_name, latest_commit_sha, "my-tag");
}
// Clean up
curl_easy_cleanup(curl);
curl_slist_free_all(headers);
json_decref(json_data);
}
}
int main() {
tag_all_repos();
return 0;
}
```
注意,上述代码仅作为示例,需要根据你的具体需求进行修改。例如,你需要修改 TODO 部分来获取每个仓库的最新提交SHA。此外,你还需要安装curl和jansson库。
阅读全文
相关推荐
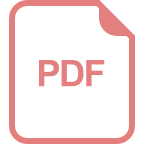
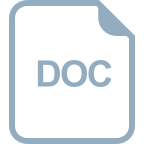
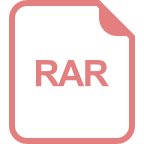
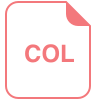
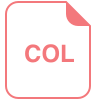
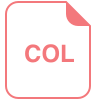
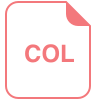
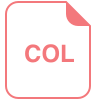
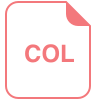

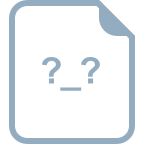