2.设计电话薄程序,根据下列描述设计类层次关系。 (1)联系人信息有:姓名、电话、备注;家人信息:还包括地址、固定电话; 朋友信息:还包括类别(同学、同事、其他朋友); (2)工作联系人信息:还包括公司、部门、职务。 (3)注重重写toString(),返回每类对象的全部信息 (4)要求在父亲中定义display()方法,此方法中将toString()结果输出值控台 (5)提供添加、删除、修改、查看指定成员、查看所有联系人信息等方法写出一个可运行的java代码
时间: 2023-07-10 17:10:39 浏览: 141
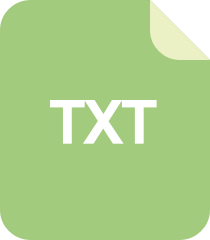
小代码,电话簿设计,程序设计周
以下是一个简单的电话薄程序的Java代码实现:
```java
import java.util.ArrayList;
import java.util.List;
import java.util.Scanner;
public class PhoneBook {
private List<Contact> contacts;
public PhoneBook() {
contacts = new ArrayList<>();
}
public void addContact(Contact contact) {
contacts.add(contact);
}
public void removeContact(int index) {
if (index >= 0 && index < contacts.size()) {
contacts.remove(index);
}
}
public void modifyContact(int index, Contact contact) {
if (index >= 0 && index < contacts.size()) {
contacts.set(index, contact);
}
}
public Contact getContact(int index) {
if (index >= 0 && index < contacts.size()) {
return contacts.get(index);
}
return null;
}
public List<Contact> getAllContacts() {
return contacts;
}
public void display() {
for (Contact contact : contacts) {
System.out.println(contact.toString());
}
}
public static void main(String[] args) {
PhoneBook phoneBook = new PhoneBook();
Scanner scanner = new Scanner(System.in);
int choice = 0;
do {
System.out.println("请选择操作:");
System.out.println("1. 添加联系人");
System.out.println("2. 删除联系人");
System.out.println("3. 修改联系人");
System.out.println("4. 查看指定联系人");
System.out.println("5. 查看所有联系人信息");
System.out.println("6. 退出程序");
System.out.print("请输入操作编号:");
choice = scanner.nextInt();
scanner.nextLine();
switch (choice) {
case 1:
System.out.print("请输入联系人类型(1:家人,2:朋友,3:工作联系人):");
int type = scanner.nextInt();
scanner.nextLine();
Contact contact = null;
switch (type) {
case 1:
System.out.print("请输入姓名:");
String name = scanner.nextLine();
System.out.print("请输入电话:");
String phone = scanner.nextLine();
System.out.print("请输入备注:");
String remark = scanner.nextLine();
System.out.print("请输入地址:");
String address = scanner.nextLine();
System.out.print("请输入固定电话:");
String fixedPhone = scanner.nextLine();
contact = new Family(name, phone, remark, address, fixedPhone);
break;
case 2:
System.out.print("请输入姓名:");
name = scanner.nextLine();
System.out.print("请输入电话:");
phone = scanner.nextLine();
System.out.print("请输入备注:");
remark = scanner.nextLine();
System.out.print("请输入类别(同学、同事、其他朋友):");
String category = scanner.nextLine();
contact = new Friend(name, phone, remark, category);
break;
case 3:
System.out.print("请输入姓名:");
name = scanner.nextLine();
System.out.print("请输入电话:");
phone = scanner.nextLine();
System.out.print("请输入备注:");
remark = scanner.nextLine();
System.out.print("请输入公司:");
String company = scanner.nextLine();
System.out.print("请输入部门:");
String department = scanner.nextLine();
System.out.print("请输入职务:");
String position = scanner.nextLine();
contact = new WorkContact(name, phone, remark, company, department, position);
break;
default:
System.out.println("输入有误,请重新输入!");
break;
}
if (contact != null) {
phoneBook.addContact(contact);
System.out.println("添加成功!");
}
break;
case 2:
System.out.print("请输入要删除的联系人编号:");
int index = scanner.nextInt();
scanner.nextLine();
phoneBook.removeContact(index);
System.out.println("删除成功!");
break;
case 3:
System.out.print("请输入要修改的联系人编号:");
index = scanner.nextInt();
scanner.nextLine();
System.out.print("请输入联系人类型(1:家人,2:朋友,3:工作联系人):");
type = scanner.nextInt();
scanner.nextLine();
switch (type) {
case 1:
System.out.print("请输入姓名:");
String name = scanner.nextLine();
System.out.print("请输入电话:");
String phone = scanner.nextLine();
System.out.print("请输入备注:");
String remark = scanner.nextLine();
System.out.print("请输入地址:");
String address = scanner.nextLine();
System.out.print("请输入固定电话:");
String fixedPhone = scanner.nextLine();
Contact newContact = new Family(name, phone, remark, address, fixedPhone);
phoneBook.modifyContact(index, newContact);
break;
case 2:
System.out.print("请输入姓名:");
name = scanner.nextLine();
System.out.print("请输入电话:");
phone = scanner.nextLine();
System.out.print("请输入备注:");
remark = scanner.nextLine();
System.out.print("请输入类别(同学、同事、其他朋友):");
String category = scanner.nextLine();
newContact = new Friend(name, phone, remark, category);
phoneBook.modifyContact(index, newContact);
break;
case 3:
System.out.print("请输入姓名:");
name = scanner.nextLine();
System.out.print("请输入电话:");
phone = scanner.nextLine();
System.out.print("请输入备注:");
remark = scanner.nextLine();
System.out.print("请输入公司:");
String company = scanner.nextLine();
System.out.print("请输入部门:");
String department = scanner.nextLine();
System.out.print("请输入职务:");
String position = scanner.nextLine();
newContact = new WorkContact(name, phone, remark, company, department, position);
phoneBook.modifyContact(index, newContact);
break;
default:
System.out.println("输入有误,请重新输入!");
break;
}
System.out.println("修改成功!");
break;
case 4:
System.out.print("请输入要查看的联系人编号:");
index = scanner.nextInt();
scanner.nextLine();
Contact targetContact = phoneBook.getContact(index);
if (targetContact != null) {
System.out.println(targetContact.toString());
} else {
System.out.println("联系人不存在!");
}
break;
case 5:
phoneBook.display();
break;
case 6:
System.out.println("退出程序,再见!");
break;
default:
System.out.println("输入有误,请重新输入!");
break;
}
} while (choice != 6);
scanner.close();
}
}
class Contact {
protected String name;
protected String phone;
protected String remark;
public Contact(String name, String phone, String remark) {
this.name = name;
this.phone = phone;
this.remark = remark;
}
@Override
public String toString() {
return "姓名:" + name + ",电话:" + phone + ",备注:" + remark;
}
}
class Family extends Contact {
private String address;
private String fixedPhone;
public Family(String name, String phone, String remark, String address, String fixedPhone) {
super(name, phone, remark);
this.address = address;
this.fixedPhone = fixedPhone;
}
@Override
public String toString() {
return super.toString() + ",地址:" + address + ",固定电话:" + fixedPhone;
}
}
class Friend extends Contact {
private String category;
public Friend(String name, String phone, String remark, String category) {
super(name, phone, remark);
this.category = category;
}
@Override
public String toString() {
return super.toString() + ",类别:" + category;
}
}
class WorkContact extends Contact {
private String company;
private String department;
private String position;
public WorkContact(String name, String phone, String remark, String company, String department, String position) {
super(name, phone, remark);
this.company = company;
this.department = department;
this.position = position;
}
@Override
public String toString() {
return super.toString() + ",公司:" + company + ",部门:" + department + ",职务:" + position;
}
}
```
阅读全文
相关推荐
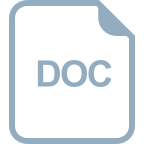
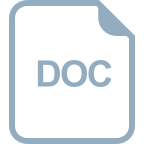
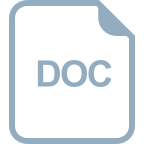
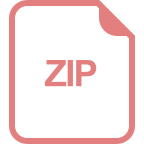
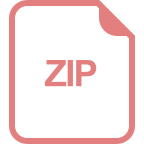
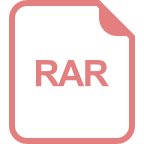
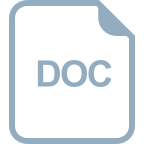
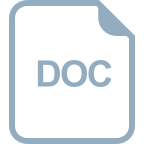
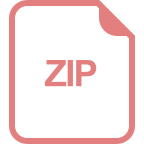
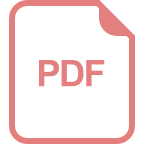
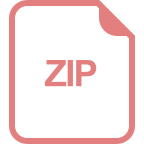
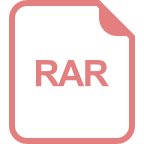
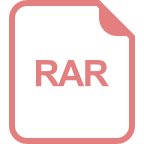
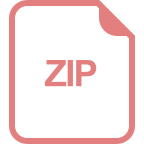
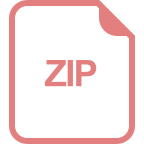
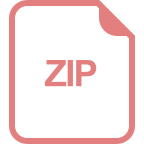