用c语言编写代码完成以下功能 按以下步骤对链表做基本操作,每一个问题输出一行 (1)创建一个链表,按顺序输入链表,并按顺序输出,以空格区分 (2)输出链表的第a个元素(从1开始计数) (3)在上一步链表的基础上,在第b个元素之前插入元素c,并输出整个链表,以空格区分 (4)在上一步链表的基础上,删除第d个元素,并输出整个链表,以空格区分 (5)在上一步链表的基础上,返回元素m第一次出现的位置,(从1开始计数)
时间: 2023-06-14 15:05:46 浏览: 74
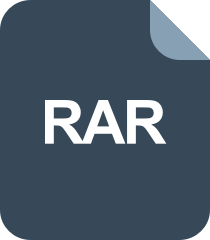
c语言实现链表的基本操作,链表是一种物理存储单元上非连续、非顺序的存储结构,数据元素的逻辑顺序是通过链表中的指针链接次序实现的。
```c
#include <stdio.h>
#include <stdlib.h>
// 链表结构体
struct Node {
int data;
struct Node *next;
};
// 创建链表
struct Node* createList() {
int num;
struct Node *head, *tail, *newNode;
head = tail = NULL;
printf("请输入链表元素,以-1结束:\n");
while (1) {
scanf("%d", &num);
if (num == -1) break;
newNode = (struct Node*) malloc(sizeof(struct Node));
newNode->data = num;
newNode->next = NULL;
if (head == NULL) {
head = tail = newNode;
} else {
tail->next = newNode;
tail = tail->next;
}
}
return head;
}
// 输出整个链表
void printList(struct Node* head) {
struct Node *p;
p = head;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
// 输出链表的第a个元素
void printNode(struct Node* head, int a) {
struct Node *p;
p = head;
for (int i = 1; i < a; i++) {
p = p->next;
}
printf("%d\n", p->data);
}
// 在第b个元素之前插入元素c
struct Node* insertNode(struct Node* head, int b, int c) {
struct Node *p, *newNode;
p = head;
for (int i = 1; i < b - 1; i++) {
p = p->next;
}
newNode = (struct Node*) malloc(sizeof(struct Node));
newNode->data = c;
if (b == 1) {
newNode->next = head;
head = newNode;
} else {
newNode->next = p->next;
p->next = newNode;
}
printList(head);
return head;
}
// 删除第d个元素
struct Node* deleteNode(struct Node* head, int d) {
struct Node *p, *q;
p = head;
if (d == 1) {
head = head->next;
free(p);
} else {
for (int i = 1; i < d - 1; i++) {
p = p->next;
}
q = p->next;
p->next = q->next;
free(q);
}
printList(head);
return head;
}
// 返回元素m第一次出现的位置
int findNode(struct Node* head, int m) {
struct Node *p;
p = head;
int index = 1;
while (p != NULL) {
if (p->data == m) {
return index;
}
p = p->next;
index++;
}
return -1; // 没有找到
}
int main() {
int a, b, c, d, m;
struct Node *head;
head = createList();
printf("整个链表为:");
printList(head);
printf("请输入要输出的节点位置:");
scanf("%d", &a);
printf("第%d个节点为:", a);
printNode(head, a);
printf("请输入要插入的元素位置和值:");
scanf("%d %d", &b, &c);
printf("插入元素后的链表为:");
head = insertNode(head, b, c);
printf("请输入要删除的节点位置:");
scanf("%d", &d);
printf("删除元素后的链表为:");
head = deleteNode(head, d);
printf("请输入要查找的元素:");
scanf("%d", &m);
int index = findNode(head, m);
if (index != -1) {
printf("元素%d第一次出现的位置为:%d\n", m, index);
} else {
printf("元素%d没有出现在链表中\n", m);
}
return 0;
}
```
阅读全文
相关推荐


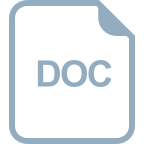
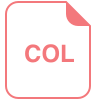








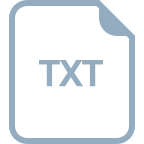
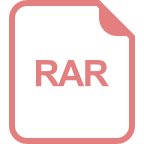
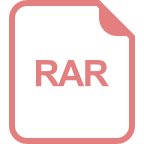
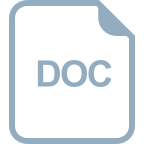