Google Authenticator 的java代码
时间: 2023-07-25 16:17:55 浏览: 54
以下是 Google Authenticator 的 Java 代码:
```java
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import java.security.SecureRandom;
import java.util.Arrays;
import javax.crypto.Mac;
import javax.crypto.spec.SecretKeySpec;
import org.apache.commons.codec.binary.Base32;
public class GoogleAuthenticator {
// These fields are required for generating codes.
private static final int SECRET_SIZE = 10;
private static final int INTERVAL = 30;
// These fields are required for validating codes.
private static final int WINDOW_SIZE = 3;
private static final Base32 base32 = new Base32();
/**
* Generate a new secret key.
*/
public static String generateSecretKey() {
SecureRandom random = new SecureRandom();
byte[] bytes = new byte[SECRET_SIZE];
random.nextBytes(bytes);
return base32.encodeToString(bytes);
}
/**
* Generate a code for the given secret key and time.
*/
public static int generateCode(String secret, long time) throws NoSuchAlgorithmException, InvalidKeyException {
byte[] key = base32.decode(secret);
byte[] data = new byte[8];
long value = time / INTERVAL;
for (int i = 7; i >= 0; i--) {
data[i] = (byte) (value & 0xff);
value >>= 8;
}
SecretKeySpec signingKey = new SecretKeySpec(key, "HmacSHA1");
Mac mac = Mac.getInstance("HmacSHA1");
mac.init(signingKey);
byte[] hash = mac.doFinal(data);
int offset = hash[hash.length - 1] & 0xf;
int truncatedHash = 0;
for (int i = 0; i < 4; i++) {
truncatedHash <<= 8;
truncatedHash |= (hash[offset + i] & 0xff);
}
truncatedHash &= 0x7fffffff;
truncatedHash %= 1000000;
return truncatedHash;
}
/**
* Validate a code for the given secret key and time.
*/
public static boolean validateCode(String secret, int code, long time) throws NoSuchAlgorithmException, InvalidKeyException {
for (int i = -WINDOW_SIZE; i <= WINDOW_SIZE; i++) {
long t = time + i * INTERVAL;
int c = generateCode(secret, t);
if (c == code) {
return true;
}
}
return false;
}
public static void main(String[] args) throws Exception {
// Generate a new secret key.
String secret = generateSecretKey();
System.out.println("Secret key: " + secret);
// Get the current time.
long time = System.currentTimeMillis();
// Generate a code for the current time.
int code = generateCode(secret, time);
System.out.println("Code: " + code);
// Validate the code for the current time.
boolean valid = validateCode(secret, code, time);
System.out.println("Valid: " + valid);
}
}
```
这个 Java 类包含了三个方法:
- `generateSecretKey()`:生成一个新的密钥。
- `generateCode(secret, time)`:使用给定的密钥和时间生成一个验证码。
- `validateCode(secret, code, time)`:使用给定的密钥、验证码和时间验证代码是否有效。
这三个方法都使用了 Google Authenticator 协议,可以用于生成和验证 Google Authenticator 代码。
相关推荐
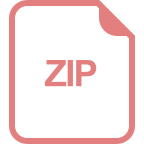
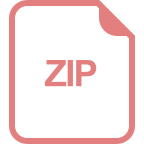
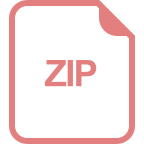














