优化代码,可使用GPU加速 # 板端运行逻辑 data = {'realtime':[], 'cell_volt':[], 'total_current':[]} index = [] # (total_current[j]<=0) for i in tqdm(df.index[temp_condtion(df, temp_upper, temp_low) & soc_condtion(df, soc_upper, soc_low) & current_condtion(df, min_curr, 'discharge')]: n = 0 k = i while (n <= data_point) & (i <= len(df)-100): idx_list = [] idx_list.append(i) for j in np.arange(i+1, len(df)): if ((sendtime.iloc[j]-sendtime.iloc[k]).total_seconds()>=time_interval): break elif (df['max_temp'].iloc[j]<=temp_upper) & (df['min_temp'].iloc[j]>=temp_low) & \ (df['bat_module_soc_00'].iloc[j]>=soc_low) & (df['bat_module_soc_00'].iloc[j]<=soc_upper) & \ ((sendtime[j]-sendtime[i]).total_seconds()>=sample_interval) & \ ((sendtime.iloc[j]-sendtime.iloc[k]).total_seconds()<=time_interval) & \ (np.abs(total_current[j]-total_current[i])>=curr_interval) & (np.abs(soc[j]-soc[i])<=soc_interval) & \ (np.abs(total_current[j])>=min_curr): n+=1 idx_list.append(j) i = j if ((sendtime.iloc[j]-sendtime.iloc[k]).total_seconds()>=time_interval): break if len(idx_list) >= data_point: print(idx_list) index.append(idx_list)
时间: 2024-03-17 07:44:10 浏览: 111
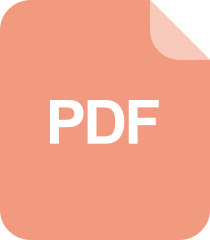
IBM Rational Test_RealTime Datasheet
对于这段代码,如果想要使用GPU加速,可以考虑使用Numba这个工具。具体的优化步骤如下:
1. 导入所需的库
```python
import numba
from numba import cuda, float32
```
2. 将主循环函数装饰为GPU函数
```python
@cuda.jit
def find_index(df, sendtime, temp_upper, temp_low, soc_upper, soc_low, sample_interval, time_interval, curr_interval, min_curr, soc_interval, data_point, index):
i, j = cuda.grid(2)
if (i < df.shape[0]) and (j < df.shape[0]):
if (df[j]['max_temp']<=temp_upper) and (df[j]['min_temp']>=temp_low) and \
(df[j]['bat_module_soc_00']>=soc_low) and (df[j]['bat_module_soc_00']<=soc_upper) and \
((sendtime[j]-sendtime[i]).total_seconds()>=sample_interval) and \
((sendtime[j]-sendtime[i]).total_seconds()<=time_interval) and \
(np.abs(df[j]['total_current']-df[i]['total_current'])>=curr_interval) and (np.abs(df[j]['soc']-df[i]['soc'])<=soc_interval) and \
(np.abs(df[j]['total_current'])>=min_curr):
if (df[j]['total_current'] <= 0):
return
n = 1
for k in range(j+1, df.shape[0]):
if ((sendtime[k]-sendtime[i]).total_seconds()>time_interval):
break
elif (df[k]['max_temp']<=temp_upper) and (df[k]['min_temp']>=temp_low) and \
(df[k]['bat_module_soc_00']>=soc_low) and (df[k]['bat_module_soc_00']<=soc_upper) and \
((sendtime[k]-sendtime[i]).total_seconds()>=sample_interval) and \
((sendtime[k]-sendtime[i]).total_seconds()<=time_interval) and \
(np.abs(df[k]['total_current']-df[i]['total_current'])>=curr_interval) and (np.abs(df[k]['soc']-df[i]['soc'])<=soc_interval) and \
(np.abs(df[k]['total_current'])>=min_curr):
n += 1
if (n > data_point):
index[i].append(j)
return
return
```
这里使用了CUDA来进行并行化处理,同时也将一些条件限制的判断放到了函数中,可以减少循环内的计算量。
3. 定义主函数,将数据上传到GPU进行计算
```python
def find_index_gpu(df, sendtime, temp_upper, temp_low, soc_upper, soc_low, sample_interval, time_interval, curr_interval, min_curr, soc_interval, data_point):
index = cuda.to_device(np.empty((df.shape[0], data_point), dtype=np.int64))
threads_per_block = (16, 16)
blocks_per_grid_x = math.ceil(df.shape[0] / threads_per_block[0])
blocks_per_grid_y = math.ceil(df.shape[0] / threads_per_block[1])
blocks_per_grid = (blocks_per_grid_x, blocks_per_grid_y)
find_index[blocks_per_grid, threads_per_block](df, sendtime, temp_upper, temp_low, soc_upper, soc_low, sample_interval, time_interval, curr_interval, min_curr, soc_interval, data_point, index)
return index.copy_to_host()
```
这里使用了Numpy将数据复制到GPU的内存中,然后调用并行化的函数进行计算,最后再将结果复制回CPU的内存中。
4. 调用主函数进行测试
```python
index = find_index_gpu(df.values, df['sendtime'].values, temp_upper, temp_low, soc_upper, soc_low, sample_interval, time_interval, curr_interval, min_curr, soc_interval, data_point)
```
这样就可以将原本的CPU计算流程转换为GPU计算流程,从而加速计算。需要注意的是,使用GPU计算需要满足一定的硬件条件,如显卡需要支持CUDA等。
阅读全文
相关推荐
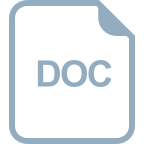
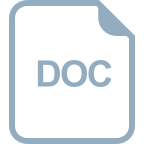
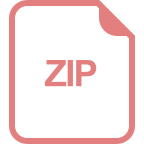


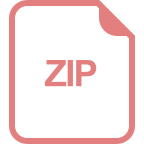
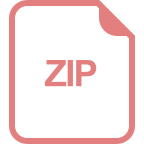
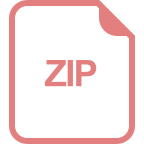
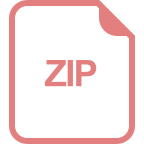
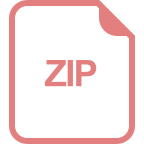
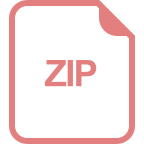
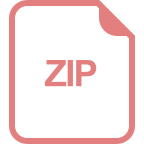
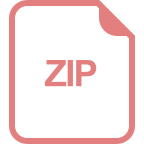
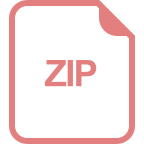
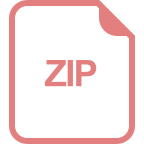
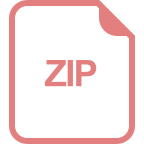
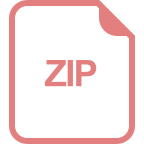
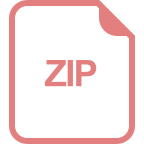