tf.nn.max_pool(input_tensor,ksize=[1,2,2,1],strides=[ 1,2,2,1], padding=""SAME"")
时间: 2024-05-25 12:13:38 浏览: 11
这是 TensorFlow 中的一个最大池化操作。它输入一个四维的张量 `input_tensor`,其中第一个维度是 batch size,第二维和第三维是输入张量的空间维度,第四维是输入张量的通道数。`ksize` 参数是一个长度为 4 的列表,表示池化窗口的大小,其中第一个和最后一个元素必须为 1,因为它们分别对应 batch size 和通道数。`strides` 参数也是一个长度为 4 的列表,表示池化窗口在每个维度上的步幅。`padding` 参数可以是 `"SAME"` 或 `"VALID"`,表示是否在边界处进行填充操作。最终输出一个四维的张量,与输入张量的第一个维度相同,第二维和第三维是池化后的空间维度,第四维是输入张量的通道数。具体来说,它会在每个池化窗口内取最大值,作为输出张量的对应位置的值。
相关问题
def avg_pool(self, bottom, name): return tf.nn.avg_pool(bottom, ksize=[1, 2, 2, 1], strides=[1, 2, 2, 1], padding='SAME', name=name) def max_pool(self, bottom, name): return tf.nn.max_pool(bottom, ksize=[1, 2, 2, 1], strides=[1, 2, 2, 1], padding='SAME', name=name) def conv_layer(self, bottom, name): with tf.variable_scope(name): filt = self.get_conv_filter(name) conv = tf.nn.conv2d(bottom, filt, [1, 1, 1, 1], padding='SAME') conv_biases = self.get_bias(name) bias = tf.nn.bias_add(conv, conv_biases) relu = tf.nn.relu(bias) return relu
这段代码定义了三个函数,用于构建VGG19模型中的卷积层和池化层。
avg_pool()函数用于定义平均池化层,其中bottom表示输入tensor,ksize表示池化窗口大小为[1, 2, 2, 1],strides表示步长为[1, 2, 2, 1],padding表示填充方式为'SAME'。
max_pool()函数用于定义最大池化层,其输入参数和参数设置与avg_pool()函数类似,只不过池化的方式不同。
conv_layer()函数用于定义卷积层,其中bottom表示输入tensor,name表示该卷积层的名称。在函数内部,首先调用了get_conv_filter()函数获取该卷积层的卷积核,然后使用tf.nn.conv2d()函数进行卷积操作,再调用get_bias()函数获取该卷积层的偏置,使用tf.nn.bias_add()函数加上偏置,最后使用ReLU激活函数激活输出结果,并将其返回。
string_tensor = tf.strings.as_string(float_tensor)咋用
`tf.strings.as_string()` 方法可以将一个数值类型的 Tensor 转换为字符串类型的 Tensor。你可以按照以下方式使用它:
```python
import tensorflow as tf
# 定义一个 float32 类型的 Tensor
float_tensor = tf.constant(3.14)
# 将 float32 类型的 Tensor 转换为字符串类型的 Tensor
string_tensor = tf.strings.as_string(float_tensor)
# 打印转换结果
tf.print(string_tensor)
```
在上面的例子中,我们首先定义了一个 `float32` 类型的 Tensor,然后使用 `tf.strings.as_string()` 方法将其转换为字符串类型的 Tensor。最后,我们使用 `tf.print()` 方法打印了转换结果。如果你想将一个 Tensor 数组中的所有元素全部转换为字符串类型,也可以使用 `tf.map_fn()` 方法来实现。
相关推荐
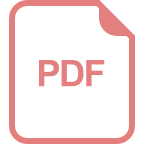














