编写代码,实现两个线程互斥运行,要求线程一完整输出1-50,线程二完整输出51-100,必须保证线程一,线程二的完整执行.用c语言写
时间: 2024-02-27 15:56:58 浏览: 22
好的,那么在C语言中,可以使用信号量来实现线程的互斥运行。下面是示例代码:
```c
#include <stdio.h>
#include <pthread.h>
#include <semaphore.h>
sem_t mutex;
void *thread_one(void *arg) {
sem_wait(&mutex);
for(int i = 1; i <= 50; i++) {
printf("%d\n", i);
}
sem_post(&mutex);
pthread_exit(NULL);
}
void *thread_two(void *arg) {
sem_wait(&mutex);
for(int i = 51; i <= 100; i++) {
printf("%d\n", i);
}
sem_post(&mutex);
pthread_exit(NULL);
}
int main() {
pthread_t t1, t2;
sem_init(&mutex, 0, 1);
pthread_create(&t1, NULL, thread_one, NULL);
pthread_create(&t2, NULL, thread_two, NULL);
pthread_join(t1, NULL);
pthread_join(t2, NULL);
sem_destroy(&mutex);
return 0;
}
```
在上面的代码中,我们使用了 `sem_t` 创建了一个信号量,并在两个线程 `thread_one` 和 `thread_two` 中分别使用了 `sem_wait()` 来等待信号量,使用了 `sem_post()` 来释放信号量。这样就保证了两个线程的互斥执行。
在主程序中,我们创建了两个线程并启动它们,使用了 `pthread_join()` 来等待两个线程的执行完成。这样就保证了两个线程的完整执行。最后使用 `sem_destroy()` 销毁信号量。
相关推荐
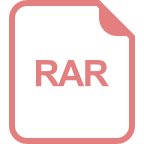
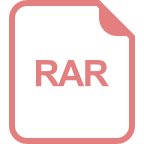















