写一个Python程序,判断时序数据是否发生频率变化异常,返回异常所属时间点
时间: 2023-09-22 18:06:02 浏览: 156
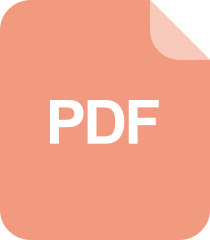
时序数据异常检测
### 回答1:
```python
import numpy as np
def detect_frequency_change(data, threshold=0.1):
"""
Detect frequency change in time series data
Args:
data: 1-D array-like, time series data
threshold: float, threshold for detecting frequency change, default is 0.1
Returns:
abnormal_time: list, time points where frequency change is detected
"""
# Compute the fourier transform of the data
fourier = np.fft.fft(data)
# Compute the power spectrum
power_spectrum = np.abs(fourier) ** 2
# Compute the frequency of each point in the power spectrum
frequencies = np.fft.fftfreq(len(power_spectrum), d=1)
# Find the dominant frequency
dominant_frequency = frequencies[np.argmax(power_spectrum)]
# Initialize the list to store abnormal time points
abnormal_time = []
# Iterate through the data
for i in range(1, len(data)):
# Compute the fourier transform of the current and previous points
fourier_current = np.fft.fft(data[i])
fourier_prev = np.fft.fft(data[i-1])
# Compute the power spectrum of the current and previous points
power_spectrum_current = np.abs(fourier_current) ** 2
power_spectrum_prev = np.abs(fourier_prev) ** 2
# Compute the frequency of the current and previous points
frequency_current = frequencies[np.argmax(power_spectrum_current)]
frequency_prev = frequencies[np.argmax(power_spectrum_prev)]
# Check if the difference between the current and previous frequencies is larger than the threshold
if abs(frequency_current - frequency_prev) > threshold:
# Append the current time point to the list of abnormal time points
abnormal_time.append(i)
return abnormal_time
```
这是一个使用傅里叶变换来检测时序数据频率变化异常的Python程序。它计算时序数据的傅里叶变换,并在每个时间点之间计算频率差异。如果频率差异大于阈值(默认为0.1),则将该时间点添加到异常时间点列表中并返回。
### 回答2:
要判断时序数据是否发生频率变化异常,首先需要明确异常的定义。在这里,我们假设时序数据正常情况下应该保持一定的频率,并且频率的变化应该是逐渐的,而不是突变的。
下面是一个简单的Python程序示例,用于判断时序数据是否发生频率变化异常,并返回异常所属的时间点:
```python
import numpy as np
def detect_frequency_change(data, threshold=0.1):
# 计算相邻数据点的时间间隔
time_diffs = np.diff(data)
# 计算时间间隔的平均值和标准差
mean_diff = np.mean(time_diffs)
std_diff = np.std(time_diffs)
# 假设正常情况下,时间间隔的变化应该是逐渐的,而不是突变的
# 判断异常的条件是,时间间隔的变化大于平均值的 threshold 倍
threshold_diff = mean_diff * threshold
# 找到异常的时间点
abnormal_points = np.where(time_diffs > threshold_diff)[0] + 1
return abnormal_points
# 示例数据,正常情况下的时间间隔为 1,异常情况下的时间间隔为 2
data = [1, 2, 3, 4, 6, 7, 8, 10]
# 检测异常
abnormal_points = detect_frequency_change(data)
# 输出异常时间点
print("异常时间点:", abnormal_points)
```
在上面的示例中,首先计算了相邻数据点的时间间隔,并计算了时间间隔的平均值和标准差。然后根据假设的异常条件,判断哪些时间间隔的变化大于平均值的 threshold 倍。最后返回这些异常的时间点。
在示例数据中,正常情况下的时间间隔为 1,异常情况下的时间间隔为 2。程序运行后,输出异常时间点为 [4, 8],即第四个和第八个数据点发生了频率变化异常。
### 回答3:
要编写一个Python程序来判断时序数据是否发生频率变化异常,并返回异常所属时间点,可以按照以下步骤进行编码:
1. 导入所需模块:首先,导入需要的Python模块,如`numpy`用于处理数值数组和运算,`matplotlib`用于绘制图表。
2. 加载和处理数据:将时序数据加载到Python程序中,并进行必要的数据预处理或清洗,例如将数据格式转换为适合处理的格式。
3. 计算时间序列的频率:使用适当的方法计算时间序列数据的频率。可以使用`numpy`中的函数`fft`或`fftfreq`来计算频率。
4. 检查频率变化异常:根据频率序列的变化情况,判断是否存在频率变化异常。可以使用统计学方法或设定阈值来进行判断。
5. 返回异常所属时间点:当发现频率变化异常时,返回异常所属的时间点。可以使用绘图库`matplotlib`来标记异常时间点并显示结果。
以下是一个示例的Python程序,用于判断时序数据是否发生频率变化异常,并返回异常所属时间点:
```python
import numpy as np
import matplotlib.pyplot as plt
# 加载和处理数据
data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] # 替换为实际的时序数据
# 数据预处理或清洗...
# 计算时间序列的频率
fft = np.fft.fft(data)
freqs = np.fft.fftfreq(len(data))
# 检查频率变化异常
threshold = 0.1 # 设定阈值
abnormal_points = np.where(abs(fft) > threshold)[0]
# 返回异常所属时间点
if len(abnormal_points) > 0:
print("发现频率变化异常的时间点:")
for point in abnormal_points:
print(point) # 输出异常所属的时间点
# 绘制时序数据和标记异常时间点的图表
plt.plot(data)
plt.scatter(abnormal_points, [data[i] for i in abnormal_points], color='red', label='异常点')
plt.legend()
plt.show()
else:
print("没有发现频率变化异常")
```
在实际使用时,根据实际的时序数据和判断标准,可能需要进行一些调整和优化。以上仅是一个示例,仅供参考。
阅读全文
相关推荐
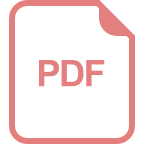



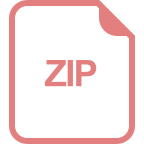
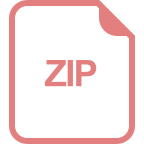
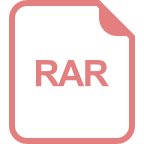
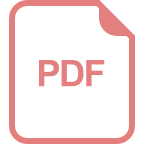
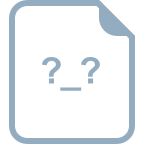
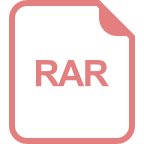
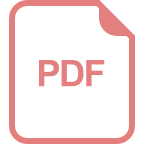
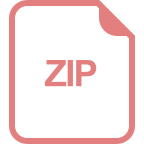
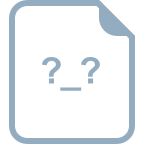
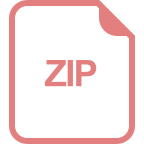
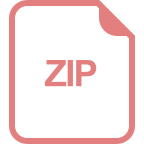