haskell实现平衡二叉树算法的代码
时间: 2023-09-15 11:05:33 浏览: 193
### 回答1:
平衡二叉树(Balanced Binary Tree)又称为 AVL 树,它是一种自平衡二叉查找树。它的性质是:任何一个节点的左子树和右子树的高度差都不超过 1。
下面是 Haskell 实现平衡二叉树的代码:
```
data Tree a = Leaf | Node (Tree a) a (Tree a) deriving (Show)
-- 计算树的高度
height :: Tree a -> Int
height Leaf = 0
height (Node l _ r) = 1 + max (height l) (height r)
-- 右旋
rightRotate :: Tree a -> Tree a
rightRotate (Node (Node a x b) y c) = Node a x (Node b y c)
rightRotate t = t
-- 左旋
leftRotate :: Tree a -> Tree a
leftRotate (Node a x (Node b y c)) = Node (Node a x b) y c
leftRotate t = t
-- 插入
insert :: (Ord a) => a -> Tree a -> Tree a
insert x Leaf = Node Leaf x Leaf
insert x (Node l y r)
| x < y = balance (Node (insert x l) y r)
| x > y = balance (Node l y (insert x r))
| otherwise = Node l y r
-- 平衡因子
balanceFactor :: Tree a -> Int
balanceFactor Leaf = 0
balanceFactor (Node l _ r) = height l - height r
-- 平衡
balance :: Tree a -> Tree a
balance t
| bf > 1 && x < y = rightRotate t
| bf < -1 && x > y = leftRotate t
| bf > 1 && x > y = leftRotate (rightRotate t)
| bf < -1 && x < y = rightRotate (leftRotate t)
| otherwise = t
where bf = balanceFactor t
x = value t
y = value (leftChild t)
-- 左子节点
leftChild :: Tree a -> Tree a
leftChild (Node l _ _) = l
-- 右子节点
rightChild :: Tree a -> Tree a
rightChild (Node _ _ r) = r
-- 节点的值
value :: Tree a -> a
value (Node _ x _) = x
-- 创建平衡二叉树
create :: (Ord a) => [a] -> Tree a
create = foldl (flip insert) Leaf
```
使用方法:
```
main = do
let t = create [3, 2, 1, 4, 5]
print t
```
输
### 回答2:
实现平衡二叉树算法的Haskell代码如下:
```
data AVLTree a = Empty | Node a Int (AVLTree a) (AVLTree a)
-- 计算树的高度
height :: AVLTree a -> Int
height Empty = 0
height (Node _ h _ _) = h
-- 计算树的平衡因子
balanceFactor :: AVLTree a -> Int
balanceFactor Empty = 0
balanceFactor (Node _ _ left right) = height left - height right
-- 左旋操作
rotateLeft :: AVLTree a -> AVLTree a
rotateLeft (Node x _ left (Node y _ middle right)) =
Node y newHeight (Node x left middle) right
where
newHeight = 1 + max (height middle) (height right)
-- 右旋操作
rotateRight :: AVLTree a -> AVLTree a
rotateRight (Node y _ (Node x _ left middle) right) =
Node x newHeight left (Node y middle right)
where
newHeight = 1 + max (height left) (height middle)
-- 平衡调整
balance :: AVLTree a -> AVLTree a
balance tree
| balanceFactor tree > 1 = rotateRight tree
| balanceFactor tree < -1 = rotateLeft tree
| otherwise = tree
-- 插入元素
insert :: Ord a => a -> AVLTree a -> AVLTree a
insert x Empty = Node x 1 Empty Empty
insert x (Node a h left right)
| x < a = balance $ Node a newHeight (insert x left) right
| x > a = balance $ Node a newHeight left (insert x right)
| otherwise = Node a h left right
where
newHeight = 1 + max (height left) (height right)
```
以上代码实现了插入操作和平衡调整操作。其中,`AVLTree` 是一个自定义的平衡二叉树类型,包含了节点的值 `a`、节点的高度 `Int`、左子树和右子树。`height` 函数用于计算树的高度,`balanceFactor` 函数用于计算树的平衡因子。`rotateLeft` 和 `rotateRight` 函数分别实现左旋和右旋操作,`balance` 函数用于调整树的平衡。`insert` 函数实现了插入操作,并通过 `balance` 函数来保持树的平衡。
### 回答3:
以下是一个简单的Haskell代码,用于实现平衡二叉树的算法:
```haskell
data Tree a = Empty | Node a (Tree a) (Tree a) deriving Show
height :: Tree a -> Int
height Empty = 0
height (Node _ left right) = 1 + max (height left) (height right)
isBalanced :: Tree a -> Bool
isBalanced Empty = True
isBalanced (Node _ left right) =
let leftHeight = height left
rightHeight = height right
in abs (leftHeight - rightHeight) <= 1 && isBalanced left && isBalanced right
-- 左旋转
rotateLeft :: Tree a -> Tree a
rotateLeft (Node val left (Node val' left' right')) =
Node val' (Node val left left') right'
-- 右旋转
rotateRight :: Tree a -> Tree a
rotateRight (Node val (Node val' left' right') right) =
Node val' left' (Node val right' right)
balance :: Tree a -> Tree a
balance Empty = Empty
balance (Node val left right)
| isBalanced (Node val left right) = Node val (balance left) (balance right)
| height left > height right = rotateRight (Node val (balance left) right)
| otherwise = rotateLeft (Node val left (balance right))
-- 示例使用
main :: IO ()
main = do
let tree = Node 1 (Node 2 (Node 3 Empty Empty) Empty) Empty
let balancedTree = balance tree
putStrLn $ "原始树:" ++ show tree
putStrLn $ "平衡树:" ++ show balancedTree
putStrLn $ "是否平衡树:" ++ show (isBalanced balancedTree)
```
该代码中定义了一个二叉树类型 `Tree`,包含了一个 `Empty` 构造子和一个 `Node` 构造子,用于构建二叉树节点。还定义了 `height` 函数用于计算树的高度,`isBalanced` 函数用于判断一个树是否是平衡二叉树。
代码中还实现了左旋转 `rotateLeft` 和右旋转 `rotateRight` 函数,以及 `balance` 函数用于将一个二叉树调整为平衡二叉树。`balance` 函数首先判断树是否已经是平衡二叉树,如果是则返回原树,否则根据左右子树的高度差进行左旋转或右旋转。
在 `main` 函数中,定义了一个示例二叉树,并将其转换为平衡二叉树,然后输出结果。
阅读全文
相关推荐
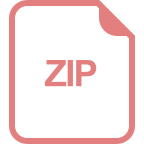














