es6中的super方法
时间: 2024-05-09 13:15:47 浏览: 8
在ES6中,super方法用于在子类中调用父类的构造函数、静态方法或原型方法。它必须在使用this关键字之前调用,否则会抛出一个ReferenceError错误。
1. 调用父类构造函数
子类必须在构造函数中调用super方法来执行父类的构造函数,以便正确地设置子类的this对象。例如:
```
class Animal {
constructor(name) {
this.name = name;
}
}
class Dog extends Animal {
constructor(name, breed) {
super(name);
this.breed = breed;
}
}
const myDog = new Dog('Buddy', 'Golden Retriever');
console.log(myDog.name); // 'Buddy'
console.log(myDog.breed); // 'Golden Retriever'
```
在这个例子中,Dog类继承自Animal类,因此它必须调用Animal类的构造函数来设置this.name属性。通过调用super(name),Dog类将传递给Animal构造函数的参数name。
2. 调用父类静态方法
子类可以调用父类的静态方法,通过使用super关键字。例如:
```
class Animal {
static sayHi() {
console.log('Hi from Animal');
}
}
class Dog extends Animal {
static sayHi() {
super.sayHi();
console.log('Hi from Dog');
}
}
Dog.sayHi(); // 'Hi from Animal' 'Hi from Dog'
```
在这个例子中,Dog类继承自Animal类,并覆盖了Animal的sayHi静态方法。通过使用super关键字,Dog类调用了Animal的sayHi静态方法,并在后面添加了自己的输出。
3. 调用父类原型方法
子类可以调用父类的原型方法,通过使用super关键字。例如:
```
class Animal {
sayHi() {
console.log('Hi from Animal');
}
}
class Dog extends Animal {
sayHi() {
super.sayHi();
console.log('Hi from Dog');
}
}
const myDog = new Dog();
myDog.sayHi(); // 'Hi from Animal' 'Hi from Dog'
```
在这个例子中,Dog类继承自Animal类,并覆盖了Animal的sayHi原型方法。通过使用super关键字,Dog类调用了Animal的sayHi方法,并在后面添加了自己的输出。
相关推荐
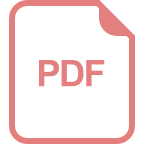
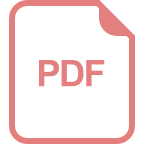














