怎么获取股票数据 python demo
时间: 2024-02-29 11:52:35 浏览: 49
你可以使用Python中的第三方库来获取股票数据,其中比较常用的有pandas-datareader和yfinance。以下是一个简单的示例代码,演示如何使用yfinance库获取股票数据:
```python
import yfinance as yf
# 获取股票数据
data = yf.download('AAPL', start='2020-01-01', end='2021-01-01')
# 打印数据
print(data)
```
在上面的代码中,我们使用yfinance库下载了苹果公司(AAPL)在2020年1月1日至2021年1月1日之间的股票数据,并将其存储在data变量中。你可以根据需要修改代码以获取其他股票的数据,或者更改start和end参数来获取不同时间范围内的数据。
相关问题
python机器人DEMO
Python机器人DEMO通常指的是使用Python编程语言构建的一个自动化脚本或程序,它模拟人类操作或完成特定任务。这类Demo可以包括各种应用场景,例如:
1. **聊天机器人**:使用自然语言处理库(如NLTK、Spacy或ChatterBot)编写能理解并回应用户输入的问题或命令的程序。
```python
from chatterbot import ChatBot
chatbot = ChatBot('My Bot')
response = chatbot.get_response('你好!')
print(response)
```
2. **Web爬虫**:使用BeautifulSoup、Scrapy等库抓取网页数据,提取信息,比如新闻、股票价格等。
```python
import requests
from bs4 import BeautifulSoup
url = 'https://www.example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
title = soup.find('h1').text
print(title)
```
3. **游戏控制**:通过Pygame等库,创建能够自动玩游戏的机器人,例如玩井字棋或扫雷。
```python
import pygame
# 简单的井字棋机器人示例
board = [[' ' for _ in range(3)] for _ in range(3)]
pygame.init()
while True:
for event in pygame.event.get():
if event.type == pygame.MOUSEBUTTONDOWN:
pos = pygame.mouse.get_pos()
row, col = pos[1] // 50, pos[0] // 50
# 添加代码处理游戏逻辑
```
使用tensorflow的lstm模型编写一个根据过往6个月股票数据预测未来18个月数据的demo
这是一个比较复杂的任务,需要一些准备工作和数据处理。
首先,我们需要获取过去6个月的股票数据和未来18个月的真实数据。这些数据可以从在线数据源或本地存储中获取。对于本教程,我们将使用 Yahoo Finance API 中的数据。
接下来,我们需要对数据进行预处理和归一化。我们将使用 MinMaxScaler 对数据进行归一化。MinMaxScaler 将数据缩放到0和1之间,这使得数据更容易处理并增加了模型的准确性。
接下来,我们将数据拆分成训练集和测试集。在本教程中,我们将使用过去90%的数据作为训练集,并将剩余10%的数据用于测试。
接下来,我们将构建一个 LSTM 模型。LSTM(Long Short-Term Memory)是一种循环神经网络,它可以处理序列数据并在长时间内记住先前的状态。在本教程中,我们将使用 TensorFlow 中的 LSTM 层来构建模型。
最后,我们将使用训练集训练模型,并使用测试集评估模型的性能。我们将使用均方根误差(RMSE)作为性能度量,因为它可以告诉我们模型的预测与实际数据之间的差异。
下面是代码实现:
```python
import numpy as np
import pandas as pd
import tensorflow as tf
from sklearn.preprocessing import MinMaxScaler
from tensorflow.keras.layers import LSTM, Dense
from tensorflow.keras.models import Sequential
# 获取数据
symbol = 'AAPL'
df = pd.read_csv(f'https://query1.finance.yahoo.com/v7/finance/download/{symbol}?period1=0&period2=9999999999&interval=1mo&events=history')
df = df[['Date', 'Close']]
df['Date'] = pd.to_datetime(df['Date'])
df = df.set_index('Date')
df = df.resample('M').last()
# 归一化数据
scaler = MinMaxScaler()
df['Close'] = scaler.fit_transform(df[['Close']])
# 拆分数据
train_size = int(len(df) * 0.9)
train_df = df[:train_size]
test_df = df[train_size:]
# 创建数据集
def create_dataset(X, y, time_steps=1):
Xs, ys = [], []
for i in range(len(X) - time_steps):
Xs.append(X.iloc[i:(i + time_steps)].values)
ys.append(y.iloc[i + time_steps])
return np.array(Xs), np.array(ys)
time_steps = 6
X_train, y_train = create_dataset(train_df, train_df['Close'], time_steps)
X_test, y_test = create_dataset(test_df, test_df['Close'], time_steps)
# 构建模型
model = Sequential([
LSTM(64, input_shape=(time_steps, X_train.shape[2])),
Dense(1)
])
model.compile(optimizer='adam', loss='mse')
# 训练模型
model.fit(
X_train, y_train,
epochs=50,
batch_size=16,
validation_split=0.1,
verbose=0,
shuffle=False
)
# 评估模型
y_pred = model.predict(X_test)
rmse = np.sqrt(tf.losses.mean_squared_error(y_test, y_pred))
print(f'RMSE: {rmse}')
# 可视化结果
import matplotlib.pyplot as plt
plt.plot(scaler.inverse_transform(y_test.reshape(-1, 1)))
plt.plot(scaler.inverse_transform(y_pred))
plt.legend(['实际', '预测'])
plt.ylabel('价格')
plt.xlabel('时间')
plt.show()
```
这段代码将获取苹果公司(AAPL)的股票数据并使用 LSTM 模型预测未来18个月的股票价格。它将使用过去6个月的数据来预测未来18个月的价格。
我们可以通过调整模型的超参数来改进模型的预测性能。例如,我们可以尝试增加 LSTM 层的数量或调整时间步长。
阅读全文
相关推荐
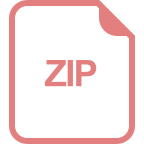
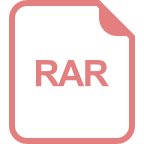
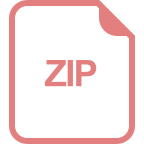
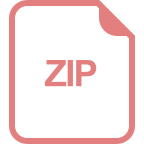
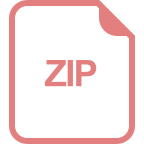
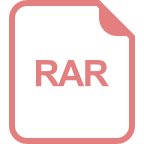
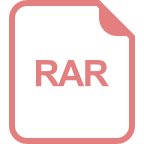
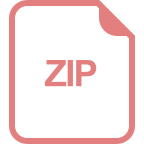
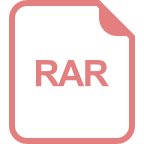
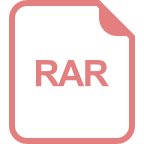
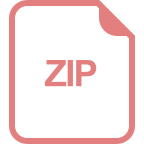
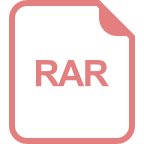
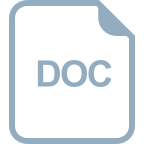
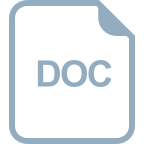