基于STM32的sim800c短信发送代码
时间: 2023-06-27 21:03:02 浏览: 842
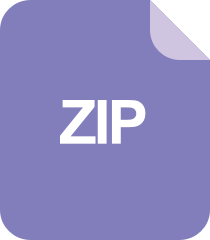
STM32+SIM800C采用HTTP协议向OneNet平台上传GPS定位数据.zip

以下是基于STM32和SIM800C模块的短信发送代码示例:
```c
#include "stm32f10x.h"
#include <stdio.h>
#include <string.h>
void USART1_Init(void);
void GPIO_Configuration(void);
void delay_ms(int ms);
int main(void)
{
char cmd[100];
USART1_Init();
GPIO_Configuration();
while (1)
{
printf("AT+CMGF=1\r\n"); //设置为文本模式
delay_ms(500);
printf("AT+CMGS=\"+86138xxxxxxxx\"\r\n"); //设置短信接收号码
delay_ms(500);
printf("Hello World!\r\n"); //设置短信内容
delay_ms(500);
printf("%c", 0x1A); //发送Ctrl+Z结束短信
delay_ms(5000);
}
}
void USART1_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_USART1, ENABLE);
//USART1_TX PA.9
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_Init(GPIOA, &GPIO_InitStructure);
//USART1_RX PA.10
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
//USART1 初始化设置
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
USART_Cmd(USART1, ENABLE); //使能串口
}
void GPIO_Configuration(void)
{
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_5;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStructure);
}
void delay_ms(int ms)
{
int i, j;
for (i = 0; i < ms; i++)
{
for (j = 0; j < 1141; j++)
{
__NOP();
}
}
}
```
在代码中,我们首先初始化了STM32的USART串口和GPIO口,然后进入一个无限循环,不断发送短信。在发送短信的过程中,我们发送了三条AT指令:
- AT+CMGF=1:将短信模式设置为文本模式。
- AT+CMGS=“+86138xxxxxxxx”:设置短信接收号码。
- Hello World!:设置短信内容。
在发送结束时,我们使用Ctrl+Z键码(0x1A)来结束短信发送。最后,我们使用延时函数来等待短信发送成功后的响应。
需要注意的是,上述代码中的号码需要替换为实际的接收号码,同时需要根据SIM800C模块的配置来调整串口波特率等参数。
阅读全文
相关推荐


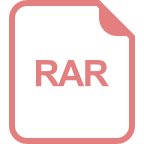



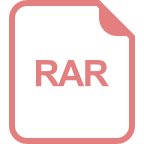




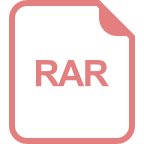
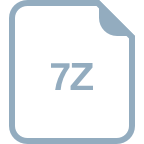
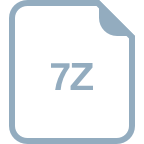
