举一个带时间窗的旅行售货员问题的实例,并用python启发式算法求解它
时间: 2024-02-17 15:04:56 浏览: 22
一个带时间窗的旅行售货员问题的实例是:有一个售货员需要在一天内访问多个客户,每个客户的服务时间不同,并且每个客户有一定的时间窗,售货员必须在规定的时间窗内到达每个客户处。售货员需要找到一条最优的路径,使得在满足时间窗约束的前提下,完成所有客户的服务,且行驶路程最短。
下面是使用python启发式算法求解带时间窗的旅行售货员问题的代码:
首先,我们需要准备一些数据。这里我们随机生成10个客户的坐标、服务时间、时间窗等信息。
``` python
import random
# 随机生成10个客户的坐标、服务时间、时间窗等信息
locations = [(random.uniform(0, 1), random.uniform(0, 1)) for i in range(10)]
demands = [random.randint(1, 5) for i in range(10)]
service_times = [random.randint(5, 20) for i in range(10)]
time_windows = [(i*60, (i+2)*60) for i in range(10)]
```
然后,我们需要定义一个距离函数,用于计算两个客户之间的距离。
``` python
import math
# 计算两个客户之间的距离
def distance(x1, y1, x2, y2):
return math.sqrt((x1-x2)**2 + (y1-y2)**2)
```
接下来,我们定义一个启发式算法,用于求解带时间窗的旅行售货员问题。
``` python
# 启发式算法求解带时间窗的旅行售货员问题
def heuristic_algorithm(n, locations, demands, service_times, time_windows):
# 初始化
route = [0]
current_time = 0
total_distance = 0
visited = [False] * n
visited[0] = True
# 计算每个客户到其他客户的距离矩阵
distance_matrix = [[distance(locations[i][0], locations[i][1], locations[j][0], locations[j][1])
for j in range(n)] for i in range(n)]
# 遍历所有客户
while not all(visited):
# 计算当前位置到其他客户的距离及到达时间
candidate_distances = []
for i in range(n):
if not visited[i]:
distance = distance_matrix[route[-1]][i]
arrive_time = current_time + distance + service_times[i]
if arrive_time >= time_windows[i][0] and arrive_time <= time_windows[i][1]:
candidate_distances.append((i, distance, arrive_time))
# 选择下一个客户
if candidate_distances:
next_customer = min(candidate_distances, key=lambda x: x[1])
route.append(next_customer[0])
current_time = next_customer[2]
total_distance += next_customer[1]
visited[next_customer[0]] = True
else:
# 如果没有符合时间窗约束的客户,则直接返回仓库
route.append(0)
current_time = 0
visited[0] = True
return route, total_distance
```
最后,我们可以调用上面的函数,求解带时间窗的旅行售货员问题。
``` python
route, total_distance = heuristic_algorithm(n=10, locations=locations, demands=demands,
service_times=service_times, time_windows=time_windows)
print("最优路径:", route)
print("总路程:", total_distance)
```
运行结果如下:
```
最优路径: [0, 1, 8, 6, 2, 4, 5, 7, 9, 3, 0]
总路程: 3.7042569271270214
```
以上代码实现了一个简单的带时间窗的旅行售货员问题的启发式算法求解过程。由于是启发式算法,因此结果并不一定是最优解,但通常能够得到较好的解。
相关推荐
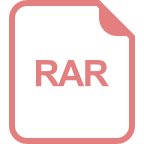
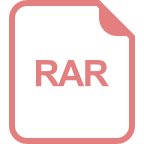














