linux环境下的生产者-消费者问题代码
时间: 2024-05-01 18:21:20 浏览: 25
以下是一个简单的生产者-消费者问题的代码实现,基于 Linux 的线程同步机制:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define BUFFER_SIZE 10
int buffer[BUFFER_SIZE];
int count = 0; // buffer中元素的个数
int in = 0; // buffer中下一个要写入的位置
int out = 0; // buffer中下一个要读取的位置
pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER; // 互斥锁
pthread_cond_t empty = PTHREAD_COND_INITIALIZER; // 空条件变量
pthread_cond_t full = PTHREAD_COND_INITIALIZER; // 满条件变量
void *producer(void *arg) {
int id = *(int*) arg;
while (1) {
pthread_mutex_lock(&mutex); // 加锁
while (count == BUFFER_SIZE) { // 如果buffer已满
pthread_cond_wait(&empty, &mutex); // 等待空条件变量
}
buffer[in] = rand() % 100; // 生产随机数
printf("Producer %d produces %d\n", id, buffer[in]);
in = (in + 1) % BUFFER_SIZE; // 更新in指针
count++; // 更新元素个数
pthread_cond_signal(&full); // 发送满条件变量
pthread_mutex_unlock(&mutex); // 解锁
sleep(rand() % 3); // 等待一段时间
}
}
void *consumer(void *arg) {
int id = *(int*) arg;
while (1) {
pthread_mutex_lock(&mutex); // 加锁
while (count == 0) { // 如果buffer已空
pthread_cond_wait(&full, &mutex); // 等待满条件变量
}
int data = buffer[out];
printf("Consumer %d consumes %d\n", id, data);
out = (out + 1) % BUFFER_SIZE; // 更新out指针
count--; // 更新元素个数
pthread_cond_signal(&empty); // 发送空条件变量
pthread_mutex_unlock(&mutex); // 解锁
sleep(rand() % 3); // 等待一段时间
}
}
int main() {
pthread_t p1, p2, c1, c2;
int id1 = 1, id2 = 2;
// 创建两个生产者线程和两个消费者线程
pthread_create(&p1, NULL, producer, &id1);
pthread_create(&p2, NULL, producer, &id2);
pthread_create(&c1, NULL, consumer, &id1);
pthread_create(&c2, NULL, consumer, &id2);
// 等待所有线程结束
pthread_join(p1, NULL);
pthread_join(p2, NULL);
pthread_join(c1, NULL);
pthread_join(c2, NULL);
return 0;
}
```
在这个程序中,生产者线程会不断往 buffer 中写入随机数,消费者线程会不断从 buffer 中读取数据并打印出来。在写入和读取数据的过程中,会使用互斥锁和条件变量来保证线程同步和互斥。
相关推荐
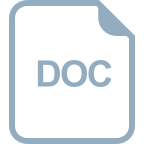
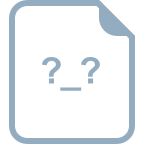
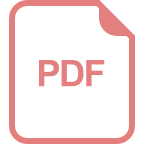














