html四则运算练习程序 假设: (1)每道练习题仅由两个数和一个运算符构成; (2)程序只能练习加减乘除四种运算; (3)每道练习题中的数满足以下要求: 在加法练习题中,两个数均为一位数; 在减法练习题中,被减数不小于减数,减数和差均为一位数; 在乘法练习题中,两个数均为一位数; 在除法练习题中,除数不为零,商为整数,除数和商均为一位数; (4)每次随机出一道练习题,用户输入答案后,判断答题是否正确; (5)答题完毕,自动出下一道练习题; (6)统计答题情况:答对的题数 / 答题数。
时间: 2023-06-15 20:05:28 浏览: 54
以下是一个简单的 HTML + JavaScript 实现:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>四则运算练习</title>
</head>
<body>
<h1>四则运算练习</h1>
<p id="question"></p>
<form>
<input type="text" id="answer">
<button type="submit">提交</button>
</form>
<p id="result"></p>
<script>
// 生成随机的练习题
function generateQuestion() {
var operators = ['+', '-', '*', '/'];
var operator = operators[Math.floor(Math.random() * operators.length)];
var a, b, answer;
switch (operator) {
case '+':
a = Math.floor(Math.random() * 10);
b = Math.floor(Math.random() * 10);
answer = a + b;
break;
case '-':
a = Math.floor(Math.random() * 10);
b = Math.floor(Math.random() * a);
answer = a - b;
break;
case '*':
a = Math.floor(Math.random() * 10);
b = Math.floor(Math.random() * 10);
answer = a * b;
break;
case '/':
b = Math.floor(Math.random() * 9) + 1;
answer = Math.floor(Math.random() * 9) + 1;
a = b * answer;
break;
}
return {a: a, b: b, operator: operator, answer: answer};
}
var question = generateQuestion();
var count = 0;
var correct = 0;
document.getElementById('question').textContent = question.a + ' ' + question.operator + ' ' + question.b;
document.querySelector('form').addEventListener('submit', function(event) {
event.preventDefault();
var userAnswer = parseInt(document.getElementById('answer').value);
if (userAnswer === question.answer) {
document.getElementById('result').textContent = '回答正确!';
correct++;
} else {
document.getElementById('result').textContent = '回答错误!正确答案是 ' + question.answer;
}
count++;
question = generateQuestion();
document.getElementById('question').textContent = question.a + ' ' + question.operator + ' ' + question.b;
document.getElementById('answer').value = '';
document.getElementById('answer').focus();
document.getElementById('stats').textContent = '答对 ' + correct + ' 题,共 ' + count + ' 题。';
});
</script>
<p id="stats"></p>
</body>
</html>
```
代码解释:
1. `generateQuestion()` 函数用于生成随机的练习题,根据运算符的不同,生成不同的数,并计算出正确答案。生成的练习题以对象的形式返回,包括两个数、一个运算符和正确答案。
2. 初始化一个练习题和答题统计变量。
3. 监听表单的提交事件,在提交时获取用户输入的答案,判断是否正确,并更新答题统计变量和下一道练习题。同时清空输入框并将光标移回输入框。
4. 在页面上显示练习题、输入框、答案提示和答题统计。
5. CSS 样式可以自行添加,例如让输入框和按钮排成一行,让答案提示突出显示等。
相关推荐
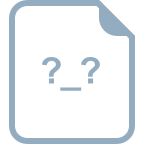
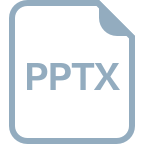














