fortran读取文件的路径
时间: 2023-07-18 10:34:39 浏览: 258
在 Fortran 中读取文件的路径可以通过指定文件名的方式来实现,例如:
```
OPEN(UNIT=1, FILE='path/to/myfile.txt', STATUS='OLD')
```
在上面的例子中,`FILE` 参数指定了文件的路径,可以根据实际情况修改为具体的路径。注意,在 Windows 系统下,路径分隔符应为反斜杠(\)而不是正斜杠(/)。
相关问题
fortran读取文本文件
Fortran是一种主要用于数值计算的编程语言,读取文本文件通常通过I/O(Input/Output)操作来完成。以下是一个基本的例子,展示了如何使用Fortran来读取文本文件中的数据:
```fortran
program read_text_file
implicit none
character(len=80) :: filename ! 文件名变量
integer :: unit_id ! 文件单位标识符
integer :: i ! 计数器
real :: value ! 存储从文件读取的数据
! 定义文件名
filename = 'example.txt'
! 打开文件,如果文件不存在则创建
open(newunit=unit_id, file=filename, status='new', action='read')
! 检查文件是否成功打开
if (iostat .ne. 0) then
write(*,*) "Error opening the file!"
stop
endif
! 使用循环逐行读取文件内容
do i = 1, 100 ! 假设你想读取前100行
read(unit_id, *) value ! 假设每行都是一个浮点数
! 对读取到的数据做处理...
print *, "Value:", value
end do
! 关闭文件
close(unit_id)
end program read_text_file
```
在这个例子中,`open`函数用于打开文件,`read`函数用于读取每一行的内容(假设为数字),然后你可以对这些数据进行处理。注意实际文件路径需要替换为你的文件位置。
Fortran如何创建文件file
在 Fortran 中,你可以使用 I/O 相关的功能来创建和操作文件。这里是一个基本的例子,展示如何使用 `unit` 变量和 `open` 操作来创建一个新的文本文件:
```fortran
program create_file
implicit none
integer :: unit ! 文件处理单位 (I/O unit)
character(len=256) :: filename ! 存储文件路径和名称
! 设置文件名
filename = 'example.txt'
! 开始一次新的 I/O 执行单元 (例如一个文件)
unit = 10 ! 使用 10 这个数值作为例子,通常会选择未使用的较大值
inquire(unit=unit, opened=file_exists) ! 判断该位置是否已分配给其他文件
if (.not. file_exists) then
open(newunit=unit, file=filename, access='write', form='formatted') ! 创建新文件并指定访问模式和文件形式
write(unit, *) 'Hello, this is a new file created in Fortran!' ! 写入一些内容
close(unit) ! 关闭文件
else
print *, 'File already exists at the specified location.'
end if
contains
logical function file_exists(unit)
logical :: file_exists
inquire(unit=unit, opened=file_exists)
end function file_exists
end program create_file
```
上述代码首先尝试打开文件,如果文件不存在则创建并写入信息,最后关闭文件。`access='write'` 表示我们只有写权限,如果需要读取也可以修改为 `'readwrite'`。`form='formatted'` 表明这是一个格式化的文本文件,对于二进制数据,可以改为 `'unformatted'`。
阅读全文
相关推荐
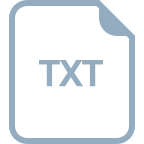
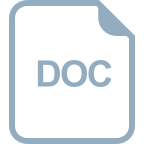
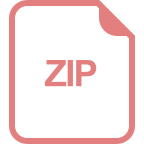
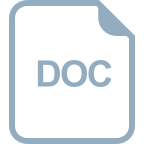

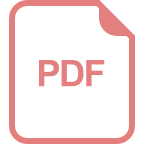
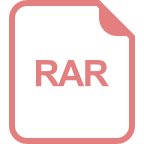
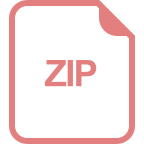
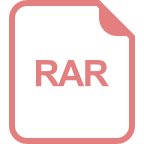
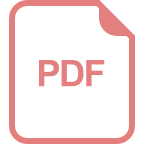
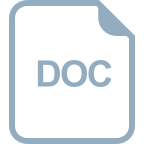

