解释const JwtStrategy = require('passport-jwt').Strategy, ExtractJwt = require('passport-jwt').ExtractJwt; const mongoose = require('mongoose') const User = mongoose.model('users') const opts = {} opts.jwtFromRequest = ExtractJwt.fromAuthHeaderAsBearerToken(); opts.secretOrKey = 'secret'; module.exports = passport =>{ passport.use(new JwtStrategy(opts, (jwt_payload, done) => { //console.log(jwt_payload) User.findById(jwt_payload.id) .then(user=>{ if(user){ return done(null, user) } return done(null, false) }) .catch(err=>console.log(err)) })) }
时间: 2024-04-28 18:25:07 浏览: 8
这是一个使用Passport和JSON Web Token (JWT)进行身份验证的Node.js应用程序中的代码片段。
首先,我们引入了`passport-jwt`模块并声明了两个常量`JwtStrategy`和`ExtractJwt`,这是提供JWT验证策略所需的模块。
接下来,引入了`mongoose`模块,并且我们假设已经在该模块中定义了一个名为`users`的模型。然后,我们声明了一个空对象`opts`,并设置了其属性`jwtFromRequest`和`secretOrKey`。
`jwtFromRequest`用于从HTTP请求的header中提取JWT,`secretOrKey`是用于解密JWT的密钥。
在模块的最后,我们导出了一个函数,该函数使用`passport`对象并传入一个JWT验证策略。JWT验证策略使用我们之前定义的选项对象`opts`和回调函数来验证JWT。回调函数中,我们从JWT中提取用户ID,然后使用Mongoose的`findById`方法查找该用户。如果找到用户,我们使用`done`函数将其提交给Passport,否则返回一个空用户。
相关问题
const User = require('../models/User');这个是什么意思
`const User = require('../models/User');` 这行代码是在 Node.js 中导入 `User` 模型的定义。它使用了 CommonJS 的模块导入语法。
在这行代码中,`require('../models/User')` 是用于导入位于 `../models/User` 路径下的模块。这个路径是相对于当前文件的路径。通常,这种路径表示在当前文件的上一级目录中的 `models` 目录下的 `User` 模块。
`User` 模块可能是一个 Mongoose 模型,它定义了用户在数据库中的结构和行为。通过导入 `User` 模块,你可以在当前文件中使用 `User` 对象来执行与用户相关的操作,如创建新用户、查询用户信息等。
请确保你在项目中正确安装了相关的模块(如 Mongoose),并且在指定的路径下存在 `User` 模块。
如果你需要进一步了解有关模块导入和导出的内容,可以查阅 Node.js 的官方文档或其他相关资源。
希望对你有所帮助!如果还有其他问题,请随时提问。
const util = require('../../utils/util.js')是什么意思
这段代码是在 Node.js 环境中引入了一个叫做 `util` 的模块,该模块是 Node.js 内置的一个工具库,包含一些常用的工具函数,如格式化时间、继承、错误处理等。`require('../../utils/util.js')` 表示该模块位于当前文件所在目录的 `utils` 子目录下,并且该子目录又在当前文件所在目录的父级目录下。
相关推荐
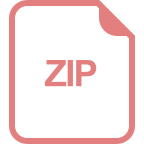
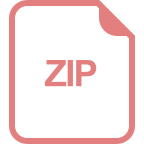
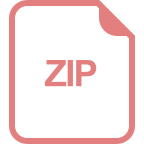












