用jieba分词进行数据预处理
时间: 2023-09-11 16:04:01 浏览: 42
首先需要安装jieba分词包,可以使用以下命令进行安装:
```
pip install jieba
```
安装完成后,就可以开始使用jieba进行数据预处理了,具体步骤如下:
1. 导入jieba包
```python
import jieba
```
2. 加载停用词表
停用词表是指一些常用的词语,如“的”、“是”、“在”等,在文本处理时需要过滤掉,以避免对模型的影响。可以从网上下载停用词表,或者自己制定。
```python
stopword_set = set()
with open('stopwords.txt','r',encoding='utf-8') as stopwords:
for stopword in stopwords:
stopword_set.add(stopword.strip('\n'))
```
3. 分词
```python
text = "这是一段需要分词的文本"
# 精确分词
seg_list = jieba.cut(text, cut_all=False)
# 去除停用词
seg_list = [word for word in seg_list if word not in stopword_set]
# 将分词结果转化为字符串
text = ' '.join(seg_list)
```
4. 批量处理
```python
import pandas as pd
# 读取数据
data = pd.read_csv('data.csv')
# 批量分词
for i in range(len(data)):
text = data['text'][i]
seg_list = jieba.cut(text, cut_all=False)
seg_list = [word for word in seg_list if word not in stopword_set]
text = ' '.join(seg_list)
data['text'][i] = text
# 保存数据
data.to_csv('data_processed.csv', index=False)
```
以上就是使用jieba分词进行数据预处理的基本步骤。需要注意的是,jieba分词是针对中文文本的,对于英文文本需要使用其他的分词工具。
相关推荐
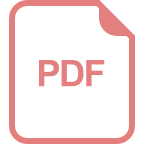
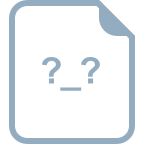





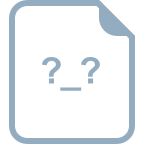
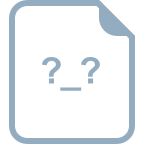
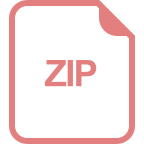
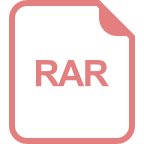
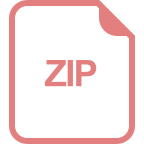
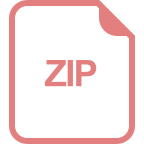
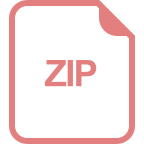