用Python实现文献Tania, S., Murshed, M., Teng, S. W., & Karmakar, G. (2020). An Enhanced Local Texture Descriptor for Image Segmentation. 2020 IEEE International Conference on Image Processing (ICIP). doi:10.1109/icip40778.2020.9190895的算法
时间: 2023-12-03 16:45:56 浏览: 28
这篇文献提出了一种改进的局部纹理描述符,可以用于图像分割。下面是用Python实现该算法的基本步骤:
1. 导入必要的库
```
import cv2
import numpy as np
from scipy.stats import skew, kurtosis
```
2. 定义函数来计算图像的标准差、偏度和峰度
```
def compute_statistics(image):
std_dev = np.std(image)
skewness = skew(image.reshape(-1))
kurt = kurtosis(image.reshape(-1))
return std_dev, skewness, kurt
```
3. 定义函数来计算局部纹理描述符
```
def compute_lbp_feature(image, radius, neighbors):
lbp = np.zeros_like(image)
for i in range(neighbors):
x = radius * np.cos(2 * np.pi * i / neighbors)
y = -radius * np.sin(2 * np.pi * i / neighbors)
x = int(round(x))
y = int(round(y))
lbp += (image > np.roll(image, -y, axis=0) - x) * (2 ** i)
hist = np.histogram(lbp, bins=range(2 ** neighbors + 1))[0].astype('float32')
hist /= sum(hist)
return hist
```
4. 定义函数来计算每个像素的局部纹理描述符
```
def compute_local_texture_descriptor(image, radius, neighbors):
pad_image = cv2.copyMakeBorder(image, radius, radius, radius, radius, cv2.BORDER_REPLICATE)
descriptor = np.zeros_like(image, dtype=np.float32)
for i in range(radius, pad_image.shape[0] - radius):
for j in range(radius, pad_image.shape[1] - radius):
patch = pad_image[i-radius:i+radius+1, j-radius:j+radius+1]
std_dev, skewness, kurt = compute_statistics(patch)
lbp_feature = compute_lbp_feature(patch, radius, neighbors)
descriptor[i-radius, j-radius] = np.concatenate(([std_dev, skewness, kurt], lbp_feature))
return descriptor
```
5. 加载图像并应用局部纹理描述符
```
image = cv2.imread('image.jpg', cv2.IMREAD_GRAYSCALE)
descriptor = compute_local_texture_descriptor(image, radius=3, neighbors=8)
```
在这个例子中,使用半径为3的LBP算子计算每个像素的局部纹理描述符。可以根据具体应用场景调整参数。
相关推荐
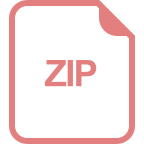
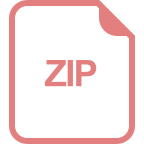
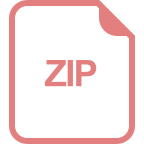
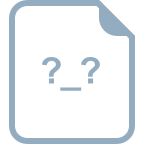
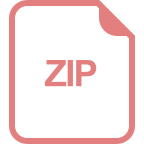
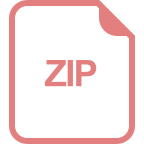
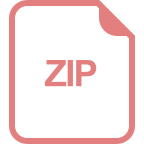
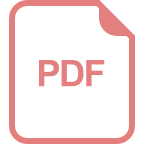
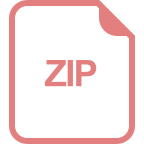
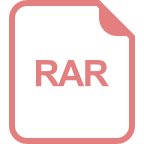
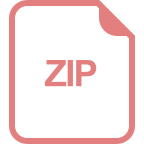