使用Tensorflow构建一个简单神经网络识别MNIST(数据集已经提前下载好并放在同级文件夹)
时间: 2024-01-25 07:02:02 浏览: 21
好的,我来给你讲解一下如何使用TensorFlow构建一个简单神经网络来识别MNIST数据集。
首先,我们需要导入需要用到的库和加载MNIST数据集。代码如下:
```python
import tensorflow as tf
from tensorflow.examples.tutorials.mnist import input_data
mnist = input_data.read_data_sets("MNIST_data/", one_hot=True)
```
接下来,我们定义神经网络的输入、输出和隐藏层的节点数。代码如下:
```python
# 输入层节点数
input_nodes = 784
# 输出层节点数
output_nodes = 10
# 隐藏层节点数
hidden_nodes = 500
```
然后,我们定义神经网络的权重和偏置变量。代码如下:
```python
# 定义权重和偏置变量
weights = {
'hidden': tf.Variable(tf.random_normal([input_nodes, hidden_nodes])),
'output': tf.Variable(tf.random_normal([hidden_nodes, output_nodes]))
}
biases = {
'hidden': tf.Variable(tf.random_normal([hidden_nodes])),
'output': tf.Variable(tf.random_normal([output_nodes]))
}
```
接下来,我们定义输入数据和输出数据的占位符。代码如下:
```python
# 定义输入数据和输出数据的占位符
x = tf.placeholder(tf.float32, [None, input_nodes])
y = tf.placeholder(tf.float32, [None, output_nodes])
```
然后,我们定义神经网络的前向传播过程。代码如下:
```python
# 定义神经网络的前向传播过程
hidden_layer = tf.nn.relu(tf.add(tf.matmul(x, weights['hidden']), biases['hidden']))
output_layer = tf.add(tf.matmul(hidden_layer, weights['output']), biases['output'])
```
接下来,我们定义损失函数和优化器。代码如下:
```python
# 定义损失函数和优化器
loss = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits(logits=output_layer, labels=y))
optimizer = tf.train.AdamOptimizer().minimize(loss)
```
最后,我们定义训练过程和评估过程,然后开始训练和评估模型。代码如下:
```python
# 定义训练过程和评估过程
correct_prediction = tf.equal(tf.argmax(output_layer, 1), tf.argmax(y, 1))
accuracy = tf.reduce_mean(tf.cast(correct_prediction, tf.float32))
# 开始训练和评估模型
with tf.Session() as sess:
sess.run(tf.global_variables_initializer())
for epoch in range(30):
avg_loss = 0.
total_batch = int(mnist.train.num_examples / 100)
for i in range(total_batch):
batch_x, batch_y = mnist.train.next_batch(100)
_, c = sess.run([optimizer, loss], feed_dict={x: batch_x, y: batch_y})
avg_loss += c / total_batch
print("Epoch:", '%04d' % (epoch + 1), "loss=", "{:.9f}".format(avg_loss))
print("Accuracy:", accuracy.eval({x: mnist.test.images, y: mnist.test.labels}))
```
以上就是使用TensorFlow构建一个简单神经网络识别MNIST的过程。希望对你有所帮助!
相关推荐
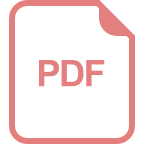
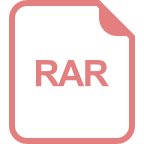














