camel 3.20.0 xml 动态循环路由合并循环结果实现
时间: 2023-07-12 09:43:42 浏览: 219
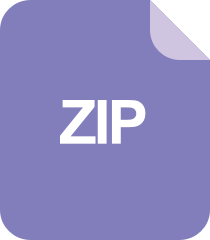
xml 循环输出

好的,下面我会给出一个简单的示例来说明如何在 Camel 3.20.0 中实现动态循环路由并合并循环结果。
首先,假设我们有一个 XML 文件如下:
```xml
<root>
<item id="1">Item 1</item>
<item id="2">Item 2</item>
<item id="3">Item 3</item>
</root>
```
我们需要使用 Camel 对每个 `<item>` 节点进行处理,并将处理结果合并为一个循环结果。
以下是一个实现该功能的路由示例:
```java
import org.apache.camel.builder.RouteBuilder;
public class MyRouteBuilder extends RouteBuilder {
@Override
public void configure() throws Exception {
// Step 1: Split the XML file into multiple messages
from("file:/path/to/xml/file")
.split().xpath("/root/item")
.setHeader("routeId").xpath("@id", String.class) // Add route ID header
.to("direct:process-item");
// Step 2: Route messages based on route ID
from("direct:process-item")
.recipientList().xpath("bean:myBean?method=getRouteEndpoint(${header.routeId})");
// Step 3: Process messages and aggregate results
from("direct:route-1")
.setBody().constant("Processing item from Route 1") // Replace with actual processing logic
.aggregate(constant(true), new MyAggregationStrategy())
.completionSize(3)
.completionTimeout(5000)
.to("log:output");
from("direct:route-2")
.setBody().constant("Processing item from Route 2") // Replace with actual processing logic
.aggregate(constant(true), new MyAggregationStrategy())
.completionSize(3)
.completionTimeout(5000)
.to("log:output");
from("direct:route-3")
.setBody().constant("Processing item from Route 3") // Replace with actual processing logic
.aggregate(constant(true), new MyAggregationStrategy())
.completionSize(3)
.completionTimeout(5000)
.to("log:output");
}
private static class MyAggregationStrategy implements AggregationStrategy {
@Override
public Exchange aggregate(Exchange oldExchange, Exchange newExchange) {
if (oldExchange == null) {
return newExchange; // First message
} else {
String oldBody = oldExchange.getIn().getBody(String.class);
String newBody = newExchange.getIn().getBody(String.class);
String mergedBody = oldBody + "\n" + newBody;
oldExchange.getIn().setBody(mergedBody);
return oldExchange;
}
}
}
public static class MyBean {
public String getRouteEndpoint(String routeId) {
return "direct:route-" + routeId;
}
}
}
```
这个路由包含了以下步骤:
1. 使用 Splitter EIP 将 XML 文件中的 `<item>` 节点拆分为多个消息,并为每个消息添加一个路由 ID 头部。
2. 使用 Recipient List EIP 根据路由 ID 将消息发送到不同的目标路由中。
3. 在目标路由中,使用 Aggregator EIP 将接收到的消息合并为一个循环结果。
在这个示例中,我们定义了三个目标路由(`direct:route-1`,`direct:route-2`,`direct:route-3`),它们的处理逻辑可以替换为您实际的处理逻辑。在每个目标路由中,我们使用 Aggregator EIP 将接收到的消息合并为一个循环结果,并使用 completionSize 和 completionTimeout 属性来控制循环结果的完成条件。在 Aggregator EIP 中,我们使用自定义的聚合策略类 `MyAggregationStrategy` 来合并消息。
最后,我们使用一个简单的 Java 类 `MyBean` 来根据路由 ID 返回目标路由的 Endpoint URI。
希望这个示例对您有所帮助!如果您有任何问题,请随时提问。
阅读全文
相关推荐
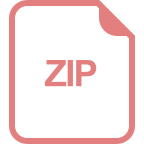
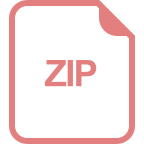

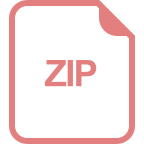
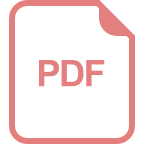
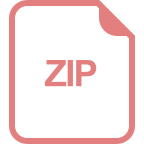
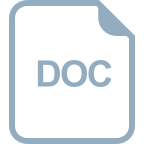
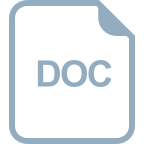