利用web前端编写一段网吧管理系统的设备管理代码
时间: 2023-08-23 13:05:48 浏览: 71
下面是一个简单的网吧设备管理系统的前端代码示例,包括设备列表展示、设备添加和设备删除功能:
```html
<!DOCTYPE html>
<html>
<head>
<title>网吧设备管理系统</title>
<meta charset="utf-8">
<style>
table {
border-collapse: collapse;
width: 100%;
}
th, td {
text-align: left;
padding: 8px;
border-bottom: 1px solid #ddd;
}
tr:hover {
background-color: #f5f5f5;
}
.button {
background-color: #4CAF50;
border: none;
color: white;
padding: 8px 16px;
text-align: center;
text-decoration: none;
display: inline-block;
font-size: 14px;
margin: 4px 2px;
cursor: pointer;
}
</style>
</head>
<body>
<h1>网吧设备管理系统</h1>
<table>
<thead>
<tr>
<th>设备名称</th>
<th>设备类型</th>
<th>设备状态</th>
<th>操作</th>
</tr>
</thead>
<tbody id="device-list">
<!-- 设备列表将动态生成 -->
</tbody>
</table>
<h2>添加设备</h2>
<form id="add-device-form">
<label for="device-name">设备名称:</label>
<input type="text" id="device-name" name="device-name" required><br>
<label for="device-type">设备类型:</label>
<select id="device-type" name="device-type">
<option value="电脑">电脑</option>
<option value="打印机">打印机</option>
<option value="投影仪">投影仪</option>
</select><br>
<button type="submit" class="button">添加设备</button>
</form>
<script>
// 获取设备列表
function getDeviceList() {
// 发送 GET 请求到后端 API 获取设备列表
fetch('/api/devices')
.then(response => response.json())
.then(devices => {
// 将设备列表渲染到页面上
const deviceList = document.querySelector('#device-list');
deviceList.innerHTML = '';
devices.forEach(device => {
const tr = document.createElement('tr');
tr.innerHTML = `
<td>${device.name}</td>
<td>${device.type}</td>
<td>${device.status}</td>
<td><button class="button" onclick="deleteDevice(${device.id})">删除</button></td>
`;
deviceList.appendChild(tr);
});
});
}
// 添加设备
const addDeviceForm = document.querySelector('#add-device-form');
addDeviceForm.addEventListener('submit', event => {
event.preventDefault();
const formData = new FormData(addDeviceForm);
// 发送 POST 请求到后端 API 添加设备
fetch('/api/devices', {
method: 'POST',
body: formData
})
.then(response => response.json())
.then(device => {
// 清空表单并重新加载设备列表
addDeviceForm.reset();
getDeviceList();
});
});
// 删除设备
function deleteDevice(deviceId) {
// 发送 DELETE 请求到后端 API 删除设备
fetch(`/api/devices/${deviceId}`, {
method: 'DELETE'
})
.then(response => response.json())
.then(result => {
// 重新加载设备列表
getDeviceList();
});
}
// 页面加载完成后立即加载设备列表
document.addEventListener('DOMContentLoaded', () => {
getDeviceList();
});
</script>
</body>
</html>
```
需要注意的是,上述代码中的设备列表、设备添加和设备删除功能均需要与后端 API 配合使用才能实现完整的网吧设备管理系统。此外,该代码示例还未对输入数据进行验证和过滤,不适用于生产环境。
相关推荐
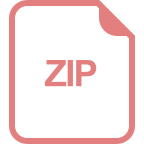
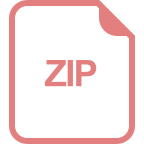
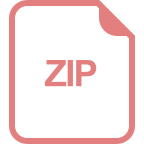














