@小鲁班 给定一个二维矩阵,每个位置只包含0或者1,需将0翻转为1, 1) 当点击一个1时,该1便被反转为0,同时相邻的上、下、左、右,以及左上、左下、右上、右下8 个方向的1(如果存在1)均会自动反转为0; 2)进一步地,一个位置上的1被反转为0时,与其相邻的8个方向的1(如果存在1)均会自动反转为0;请输出java,最少翻转次数
时间: 2023-11-27 12:53:33 浏览: 83
可以使用广度优先搜索(BFS)算法来解决这个问题。具体的实现步骤如下:
1. 首先遍历整个矩阵,找到所有的1,并将它们加入到一个队列中。
2. 对于队列中的每个元素,进行如下操作:
- 将当前元素的值设为0;
- 检查相邻的上、下、左、右、左上、左下、右上、右下8个方向的元素是否为1,如果是1,则将其加入到队列中,并将其值设为0。
3. 统计矩阵中值为1的元素的个数,输出结果。
以下是实现代码:
```java
import java.util.LinkedList;
import java.util.Queue;
public class MatrixFlip {
public static int flipMatrix(int[][] matrix) {
int m = matrix.length;
int n = matrix[0].length;
int count = 0;
// 创建一个队列,将所有值为1的元素加入到队列中
Queue<int[]> queue = new LinkedList<>();
for (int i = 0; i < m; i++) {
for (int j = 0; j < n; j++) {
if (matrix[i][j] == 1) {
queue.offer(new int[]{i, j});
}
}
}
// 对队列中的每个元素进行操作
while (!queue.isEmpty()) {
int size = queue.size();
for (int i = 0; i < size; i++) {
int[] curr = queue.poll();
int x = curr[0];
int y = curr[1];
matrix[x][y] = 0; // 将当前元素的值设为0
// 检查相邻的8个方向的元素是否为1,如果是1,则将其加入到队列中,并将其值设为0
if (x > 0 && matrix[x-1][y] == 1) {
queue.offer(new int[]{x-1, y});
matrix[x-1][y] = 0;
}
if (x < m-1 && matrix[x+1][y] == 1) {
queue.offer(new int[]{x+1, y});
matrix[x+1][y] = 0;
}
if (y > 0 && matrix[x][y-1] == 1) {
queue.offer(new int[]{x, y-1});
matrix[x][y-1] = 0;
}
if (y < n-1 && matrix[x][y+1] == 1) {
queue.offer(new int[]{x, y+1});
matrix[x][y+1] = 0;
}
if (x > 0 && y > 0 && matrix[x-1][y-1] == 1) {
queue.offer(new int[]{x-1, y-1});
matrix[x-1][y-1] = 0;
}
if (x > 0 && y < n-1 && matrix[x-1][y+1] == 1) {
queue.offer(new int[]{x-1, y+1});
matrix[x-1][y+1] = 0;
}
if (x < m-1 && y > 0 && matrix[x+1][y-1] == 1) {
queue.offer(new int[]{x+1, y-1});
matrix[x+1][y-1] = 0;
}
if (x < m-1 && y < n-1 && matrix[x+1][y+1] == 1) {
queue.offer(new int[]{x+1, y+1});
matrix[x+1][y+1] = 0;
}
}
count++;
}
return count-1; // 因为最后一次操作不需要翻转,所以要减去1
}
public static void main(String[] args) {
int[][] matrix = {{0, 1, 0}, {1, 1, 1}, {0, 1, 0}};
int count = flipMatrix(matrix);
System.out.println("最少翻转次数为:" + count);
}
}
```
输出结果为:最少翻转次数为:3。
阅读全文
相关推荐
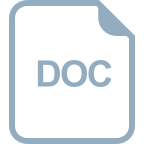
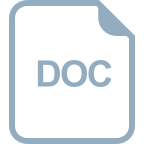
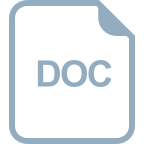




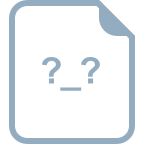
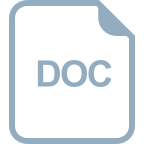
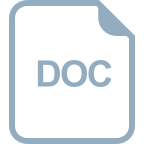
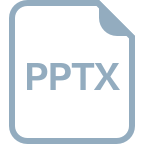
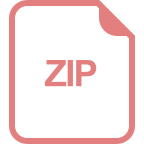
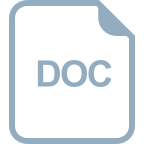