struct xinxi *duqu2() { struct xinxi *head2= NULL; struct xinxi *temp = NULL; char dianhua[20], mima[20],chepaihao[20]; FILE *fp = fopen("用户信息.txt", "r"); if (fp == NULL) { printf("\t\t\t\t打开文件失败!文件名: 用户信息.txt\n"); return NULL; } else { while (fscanf(fp, "%s %s %s", dianhua, mima,chepaihao) != EOF) { struct xinxi *p = (struct xinxi *)malloc(sizeof(struct xinxi)); if (p == NULL) { printf("分配内存失败!\n"); } strcpy(p->dianhua, dianhua); strcpy(p->mima, mima); strcpy(p->chepaihao,chepaihao); if (head2 == NULL) { head2 = temp = p; } else { temp->next = p; temp = p; } } fclose(fp); if (temp != NULL) { temp->next = NULL; } return head2; } } void chongzhi() { char dianhua[N]; char mima[N]; char xinmima[N]; struct xinxi *head2,*p,*q; p=head2=duqu2(); printf("请输入账号(联系方式):"); scanf("%s",dianhua); printf("请输入原密码:"); scanf("%s",mima); while(p!=NULL) { if(strcmp(dianhua,p->dianhua)==0&&strcmp(mima,p->mima)==0) { printf("请输入新密码:"); scanf("%s",xinmima); strcpy(p->mima,xinmima); break; } p=p->next; } if(p==NULL) { printf("\t\t\t\t账号(联系方式)或密码错误!\n"); } else { FILE *fp; fp=fopen("\t\t\t\t用户信息.txt","w"); q=head2; while(q!=NULL) { fprintf(fp,"%s %s %s\n", q->dianhua, q->mima,q->chepaihao); q=q->next; } fclose(fp); printf("\t\t\t\t重置成功!\n"); } }为什么错了
时间: 2024-04-28 22:26:36 浏览: 105
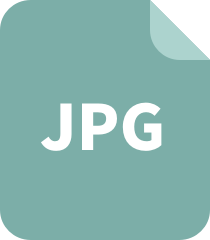
内存错误
在 `chongzhi()` 函数中,`head2` 指针没有被初始化,而是直接调用了 `duqu2()` 函数返回的空指针。因此,在 `head2` 指针未初始化的情况下,对其进行操作会导致程序崩溃或者出现意料之外的结果。
你需要在 `chongzhi()` 函数的开头,使用 `duqu2()` 函数初始化 `head2` 指针,即将 `struct xinxi *head2=NULL;` 修改为 `struct xinxi *head2=duqu2();`,这样才能对 `head2` 指针进行操作。
阅读全文
相关推荐
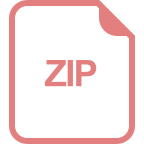




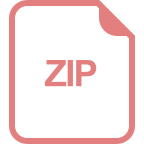
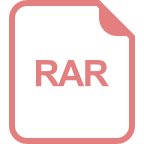
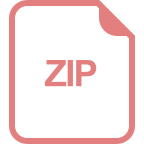
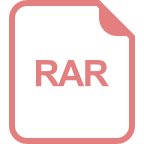
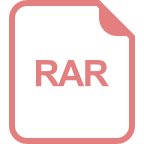
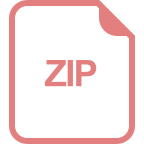
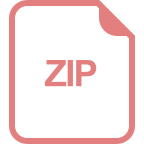
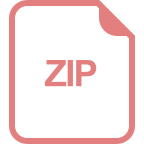