python中jsonpath模块用法
时间: 2024-06-09 10:08:27 浏览: 124
jsonpath是一个用于在JSON对象中查找、读取和过滤节点的库。它提供了一种类似XPath的语法来定位节点。
安装:
```
pip install jsonpath
```
使用示例:
假设我们有如下JSON对象:
```python
{
"store": {
"book": [
{
"category": "reference",
"author": "Nigel Rees",
"title": "Sayings of the Century",
"price": 8.95
},
{
"category": "fiction",
"author": "Evelyn Waugh",
"title": "Sword of Honour",
"price": 12.99
},
{
"category": "fiction",
"author": "Herman Melville",
"title": "Moby Dick",
"isbn": "0-553-21311-3",
"price": 8.99
},
{
"category": "fiction",
"author": "J. R. R. Tolkien",
"title": "The Lord of the Rings",
"isbn": "0-395-19395-8",
"price": 22.99
}
],
"bicycle": {
"color": "red",
"price": 19.95
}
},
"expensive": 10
}
```
1. 获取所有book节点的title属性值
```python
import json
from jsonpath import jsonpath
# 加载JSON对象
obj = json.loads('{"store":{"book":[{"category":"reference","author":"Nigel Rees","title":"Sayings of the Century","price":8.95},{"category":"fiction","author":"Evelyn Waugh","title":"Sword of Honour","price":12.99},{"category":"fiction","author":"Herman Melville","title":"Moby Dick","isbn":"0-553-21311-3","price":8.99},{"category":"fiction","author":"J. R. R. Tolkien","title":"The Lord of the Rings","isbn":"0-395-19395-8","price":22.99}],"bicycle":{"color":"red","price":19.95}},"expensive":10}')
# 获取所有book节点的title属性值
titles = jsonpath(obj, '$.store.book[*].title')
print(titles)
```
输出结果:
```
['Sayings of the Century', 'Sword of Honour', 'Moby Dick', 'The Lord of the Rings']
```
2. 获取所有价格小于10的book节点
```python
import json
from jsonpath import jsonpath
# 加载JSON对象
obj = json.loads('{"store":{"book":[{"category":"reference","author":"Nigel Rees","title":"Sayings of the Century","price":8.95},{"category":"fiction","author":"Evelyn Waugh","title":"Sword of Honour","price":12.99},{"category":"fiction","author":"Herman Melville","title":"Moby Dick","isbn":"0-553-21311-3","price":8.99},{"category":"fiction","author":"J. R. R. Tolkien","title":"The Lord of the Rings","isbn":"0-395-19395-8","price":22.99}],"bicycle":{"color":"red","price":19.95}},"expensive":10}')
# 获取所有价格小于10的book节点
books = jsonpath(obj, '$.store.book[?(@.price < 10)]')
print(books)
```
输出结果:
```
[{'category': 'reference', 'author': 'Nigel Rees', 'title': 'Sayings of the Century', 'price': 8.95}, {'category': 'fiction', 'author': 'Herman Melville', 'title': 'Moby Dick', 'isbn': '0-553-21311-3', 'price': 8.99}]
```
3. 获取所有书的作者和价格列表
```python
import json
from jsonpath import jsonpath
# 加载JSON对象
obj = json.loads('{"store":{"book":[{"category":"reference","author":"Nigel Rees","title":"Sayings of the Century","price":8.95},{"category":"fiction","author":"Evelyn Waugh","title":"Sword of Honour","price":12.99},{"category":"fiction","author":"Herman Melville","title":"Moby Dick","isbn":"0-553-21311-3","price":8.99},{"category":"fiction","author":"J. R. R. Tolkien","title":"The Lord of the Rings","isbn":"0-395-19395-8","price":22.99}],"bicycle":{"color":"red","price":19.95}},"expensive":10}')
# 获取所有书的作者和价格列表
info = jsonpath(obj, '$.store.book[*].{author: author, price: price}')
print(info)
```
输出结果:
```
[{'author': 'Nigel Rees', 'price': 8.95}, {'author': 'Evelyn Waugh', 'price': 12.99}, {'author': 'Herman Melville', 'price': 8.99}, {'author': 'J. R. R. Tolkien', 'price': 22.99}]
```
更多使用方法,可以查看jsonpath的文档。
阅读全文
相关推荐









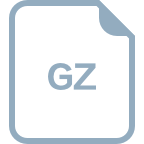







