如何使用代码批量导入excel数据到数据库
时间: 2023-05-19 12:05:18 浏览: 140
您可以使用Python中的pandas库来实现批量导入excel数据到数据库。首先,您需要安装pandas库和数据库连接器,例如pymysql或者sqlite3。然后,您可以使用pandas的read_excel函数读取excel文件,并将数据存储到pandas的DataFrame对象中。接下来,您可以使用pandas的to_sql函数将DataFrame对象中的数据批量导入到数据库中。以下是示例代码:
```python
import pandas as pd
import pymysql
# 连接数据库
conn = pymysql.connect(host='localhost', user='root', password='password', db='test')
# 读取excel文件
df = pd.read_excel('data.xlsx')
# 将数据导入到数据库
df.to_sql('table_name', conn, if_exists='replace', index=False)
# 关闭数据库连接
conn.close()
```
其中,'data.xlsx'是您要导入的excel文件名,'table_name'是您要导入的数据库表名。如果数据库中已经存在同名的表,可以使用if_exists参数来指定替换或追加数据。
相关问题
如何使用mybatis代码批量导入excel数据到数据库
您可以使用以下步骤来使用 MyBatis 代码批量导入 Excel 数据到数据库:
1. 创建一个 POJO 类来表示 Excel 表格中的每一行数据。
2. 使用 Apache POI 库来读取 Excel 文件并将数据存储到 POJO 对象中。
3. 创建一个 MyBatis Mapper 接口和对应的 XML 文件,用于将 POJO 对象插入到数据库中。
4. 在 Mapper 接口中定义一个批量插入的方法,使用 MyBatis 提供的批量操作 API 批量插入数据。
5. 在代码中调用批量插入方法,将读取到的 Excel 数据批量插入到数据库中。
以下是一个示例代码:
// 定义 POJO 类
public class ExcelData {
private String name;
private int age;
// 省略 getter 和 setter 方法
}
// 使用 Apache POI 读取 Excel 文件
List<ExcelData> dataList = new ArrayList<>();
Workbook workbook = WorkbookFactory.create(new File("data.xlsx"));
Sheet sheet = workbook.getSheetAt(0);
for (Row row : sheet) {
ExcelData data = new ExcelData();
data.setName(row.getCell(0).getStringCellValue());
data.setAge((int) row.getCell(1).getNumericCellValue());
dataList.add(data);
}
// 定义 MyBatis Mapper 接口和 XML 文件
public interface ExcelDataMapper {
void insertBatch(List<ExcelData> dataList);
}
<!-- ExcelDataMapper.xml -->
<mapper namespace="com.example.mapper.ExcelDataMapper">
<insert id="insertBatch" parameterType="java.util.List">
INSERT INTO excel_data (name, age) VALUES
<foreach collection="list" item="item" separator=",">
(#{item.name}, #{item.age})
</foreach>
</insert>
</mapper>
// 调用批量插入方法
SqlSessionFactory sessionFactory = new SqlSessionFactoryBuilder().build(inputStream);
try (SqlSession session = sessionFactory.openSession(ExecutorType.BATCH)) {
ExcelDataMapper mapper = session.getMapper(ExcelDataMapper.class);
mapper.insertBatch(dataList);
session.commit();
}
如何使用Java代码批量导入excel数据到数据库
您可以使用Apache POI库来读取Excel文件中的数据,然后使用JDBC连接到数据库并将数据插入到数据库中。以下是一个简单的示例代码:
```java
import java.io.File;
import java.io.FileInputStream;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelToDatabase {
public static void main(String[] args) {
String jdbcUrl = "jdbc:mysql://localhost:3306/mydatabase";
String username = "myusername";
String password = "mypassword";
String excelFilePath = "data.xlsx";
try (Connection connection = DriverManager.getConnection(jdbcUrl, username, password)) {
FileInputStream inputStream = new FileInputStream(new File(excelFilePath));
Workbook workbook = new XSSFWorkbook(inputStream);
Sheet sheet = workbook.getSheetAt(0);
String sql = "INSERT INTO mytable (column1, column2, column3) VALUES (?, ?, ?)";
PreparedStatement statement = connection.prepareStatement(sql);
int rowNumber = 0;
for (Row row : sheet) {
if (rowNumber == 0) {
rowNumber++;
continue;
}
Cell cell1 = row.getCell(0);
String column1 = cell1.getStringCellValue();
Cell cell2 = row.getCell(1);
String column2 = cell2.getStringCellValue();
Cell cell3 = row.getCell(2);
double column3 = cell3.getNumericCellValue();
statement.setString(1, column1);
statement.setString(2, column2);
statement.setDouble(3, column3);
statement.addBatch();
if (rowNumber % 1000 == 0) {
statement.executeBatch();
}
}
workbook.close();
statement.executeBatch();
System.out.println("Data imported successfully.");
} catch (SQLException e) {
System.out.println("Database error:");
e.printStackTrace();
} catch (Exception e) {
System.out.println("Error:");
e.printStackTrace();
}
}
}
```
请注意,此代码仅适用于Excel文件的第一个工作表,并且假定数据库中已经存在名为“mytable”的表,该表具有三个列:column1,column2和column3。您需要根据自己的需求进行修改。
相关推荐
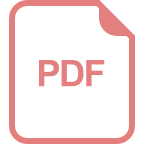
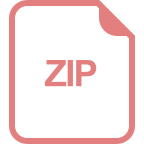












