用python语言写出来
时间: 2024-04-03 16:35:51 浏览: 117
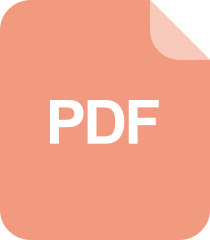
使用Python写一个小游戏
好的,我为您提供一个基于 Python+Opencv 的简单的银行卡号识别系统示例代码,仅供参考:
```python
import cv2
import numpy as np
import pytesseract
# 图像预处理
def preprocess(image):
# 灰度化
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 去噪
blur = cv2.GaussianBlur(gray, (5, 5), 0)
# 二值化
_, binary = cv2.threshold(blur, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)
# 形态学操作
kernel = cv2.getStructuringElement(cv2.MORPH_RECT, (3, 3))
morph = cv2.morphologyEx(binary, cv2.MORPH_CLOSE, kernel)
return morph
# 银行卡区域定位
def locate_card(image):
# 读取模板图像
template = cv2.imread('template.jpg', 0)
# 模板匹配
result = cv2.matchTemplate(image, template, cv2.TM_CCOEFF_NORMED)
_, max_val, _, max_loc = cv2.minMaxLoc(result)
if max_val < 0.6:
return None
# 计算银行卡区域坐标
_, _, w, h = template.shape
card_loc = (max_loc[0], max_loc[1], w, h)
return card_loc
# 文本区域定位
def locate_text(image, card_loc):
# 截取银行卡区域
x, y, w, h = card_loc
card = image[y:y+h, x:x+w]
# 文本检测
text_detector = cv2.text.TextDetectorCNN_create('textbox.prototxt', 'TextBoxes_icdar13.caffemodel')
boxes, scores = text_detector.detect(card)
# 计算卡号区域坐标
card_width = card.shape[1]
card_height = card.shape[0]
text_loc = None
for i in range(len(scores)):
if scores[i] > 0.5:
x1, y1, x2, y2 = boxes[i]
x1 = int(x1 * card_width)
y1 = int(y1 * card_height)
x2 = int(x2 * card_width)
y2 = int(y2 * card_height)
if (x2 - x1) > card_width * 0.5 and (y2 - y1) > card_height * 0.1:
text_loc = (x1 + x, y1 + y, x2 - x1, y2 - y1)
break
return text_loc
# 文本识别
def recognize_text(image, text_loc):
# 截取卡号区域
x, y, w, h = text_loc
text = image[y:y+h, x:x+w]
# 文本识别
card_num = pytesseract.image_to_string(text, config='digits')
return card_num
# 调用主函数
if __name__ == '__main__':
# 读取图像
image = cv2.imread('card.jpg')
# 图像预处理
processed = preprocess(image)
# 银行卡区域定位
card_loc = locate_card(processed)
if card_loc is None:
print("Can't find card!")
exit()
# 文本区域定位
text_loc = locate_text(processed, card_loc)
if text_loc is None:
print("Can't find text!")
exit()
# 文本识别
card_num = recognize_text(image, text_loc)
print("Card number:", card_num)
# 显示结果
cv2.rectangle(image, (card_loc[0], card_loc[1]), (card_loc[0]+card_loc[2], card_loc[1]+card_loc[3]), (0, 0, 255), 2)
cv2.rectangle(image, (text_loc[0], text_loc[1]), (text_loc[0]+text_loc[2], text_loc[1]+text_loc[3]), (0, 255, 0), 2)
cv2.putText(image, card_num, (text_loc[0], text_loc[1]-10), cv2.FONT_HERSHEY_SIMPLEX, 1, (0, 255, 0), 2)
cv2.imshow('Result', image)
cv2.waitKey(0)
```
这段代码实现了一个简单的银行卡号识别系统,具体实现流程与上面所述的大致相同,但是还有很多细节需要注意,比如模板匹配的阈值、文本检测的参数设置等。如果您需要更详细的代码实现或者有其他问题需要咨询,请随时告诉我。
阅读全文
相关推荐
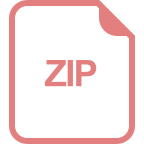
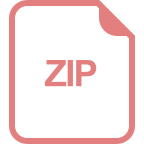
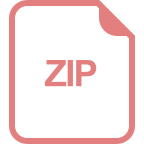
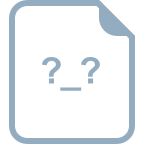
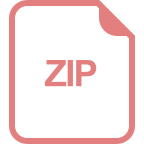
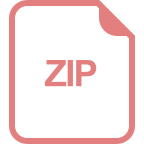
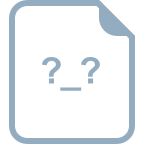
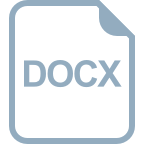
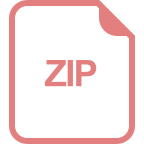