c语言由实参传来一个字符串
时间: 2023-12-26 22:05:04 浏览: 39
在C语言中,可以通过函数参数传递字符串。字符串在C语言中是以字符数组的形式表示的,因此可以将字符数组作为参数传递给函数。例如,下面的代码演示了如何通过函数参数传递字符串:
```c
#include <stdio.h>
void print_string(char *str)
{
printf("%s\n", str);
}
int main()
{
char my_string[] = "Hello, world!";
print_string(my_string);
return 0;
}
```
在上面的代码中,`print_string`函数接受一个字符指针参数`str`,并将该字符串打印到控制台上。在`main`函数中,我们定义了一个字符数组`my_string`,并将其作为参数传递给`print_string`函数。该函数将打印`"Hello, world!"`到控制台上。
相关问题
由C语言实现编写一个函数由实参传来一个字符串统计此字符串中字母数字空格和其他字符的个数在主函数中输入字符串以及输出上述的结果
下面是一个简单的C语言函数,可以统计一个字符串中字母、数字、空格和其他字符的个数:
```c
#include <stdio.h>
#include <ctype.h>
void count_chars(char *str, int *letters, int *digits, int *spaces, int *others) {
char ch;
*letters = *digits = *spaces = *others = 0;
while ((ch = *str++) != '\0') {
if (isalpha(ch))
(*letters)++;
else if (isdigit(ch))
(*digits)++;
else if (isspace(ch))
(*spaces)++;
else
(*others)++;
}
}
int main() {
char str[100];
int letters, digits, spaces, others;
printf("Enter a string: ");
fgets(str, sizeof(str), stdin);
count_chars(str, &letters, &digits, &spaces, &others);
printf("Letters: %d\n", letters);
printf("Digits: %d\n", digits);
printf("Spaces: %d\n", spaces);
printf("Other characters: %d\n", others);
return 0;
}
```
在主函数中,我们首先输入一个字符串,然后调用 `count_chars` 函数来统计字符的个数。函数使用 `ctype.h` 中的 `isalpha`、`isdigit` 和 `isspace` 函数来判断字符的类型。在函数中,我们使用指针来返回每种类型字符的个数,因为需要修改这些变量的值。在主函数中,我们只需要打印这些变量的值即可。
C语言编写一个函数,由实参传来一个字符串,统计此字符串中字母、数字、空格和其它字符的个数,在主函数中输入字符串并输出统计结果。
可以使用C语言的字符处理函数以及循环来实现这个功能。
以下是一个实现该功能的示例代码:
```c
#include <stdio.h>
#include <ctype.h>
void count_chars(char *str, int *letters, int *digits, int *spaces, int *others) {
char c;
*letters = *digits = *spaces = *others = 0;
while ((c = *str++) != '\0') {
if (isalpha(c)) {
(*letters)++;
} else if (isdigit(c)) {
(*digits)++;
} else if (isspace(c)) {
(*spaces)++;
} else {
(*others)++;
}
}
}
int main() {
char str[100];
int letters, digits, spaces, others;
printf("请输入一个字符串:\n");
fgets(str, sizeof(str), stdin);
count_chars(str, &letters, &digits, &spaces, &others);
printf("字母个数:%d\n", letters);
printf("数字个数:%d\n", digits);
printf("空格个数:%d\n", spaces);
printf("其它字符个数:%d\n", others);
return 0;
}
```
在这个代码中,`count_chars` 函数接收一个字符串指针和四个整型指针作为参数,分别用来存储字母、数字、空格和其它字符的个数。在函数中,首先将这四个指针所指向的变量初始化为 0,然后使用循环遍历字符串中的每一个字符,并根据其类型来更新对应的计数器。循环结束后,函数返回,并将统计结果存储在四个指针所指向的变量中。
在 `main` 函数中,首先使用 `fgets` 函数读取用户输入的字符串,并将其存储在 `str` 数组中。然后,调用 `count_chars` 函数统计字符串中各种字符的个数,并将结果输出到控制台。
相关推荐
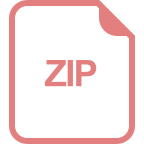
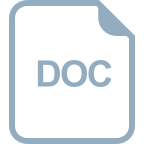












