用split来计算python中计算某个单词出现次数
时间: 2023-10-09 15:10:08 浏览: 178
可以使用Python中的split()函数和计数器Counter来计算某个单词出现的次数。具体步骤如下:
1. 读取文本文件,将其转换为字符串。
2. 使用split()函数将字符串分割成单词列表。
3. 使用Counter()函数统计单词出现次数。
4. 输出指定单词的出现次数。
以下是示例代码:
```python
from collections import Counter
# 读取文本文件,将其转换为字符串
with open('text.txt', 'r') as f:
text = f.read()
# 使用 split() 函数将字符串分割成单词列表
words = text.split()
# 使用 Counter() 函数统计单词出现次数
word_counts = Counter(words)
# 输出指定单词的出现次数
print(word_counts['python'])
```
其中,`text.txt`是需要计算单词出现次数的文本文件,`python`是需要统计的单词。
相关问题
使用split来计算python中某个特定单词出现次数
可以使用Python内置的`split()`函数和列表的计数方法来计算某个特定单词在一个字符串中出现的次数,具体步骤如下:
1. 将字符串使用`split()`函数拆分成一个单词列表。
2. 使用列表的`count()`方法统计特定单词在列表中出现的次数。
示例代码如下:
```python
string = "This is a sample string to demonstrate how to count a specific word in python"
word = "to"
word_list = string.split()
count = word_list.count(word)
print("The word '{}' appears {} times in the string.".format(word, count))
```
输出结果为:
```
The word 'to' appears 2 times in the string.
```
注意:这种方式只能计算完全匹配的单词,如果需要计算包含该单词的子串的情况,需要使用正则表达式等其他方法。
使用splitpython中计算某个单词出现次数
可以使用Python中的`split()`函数和字典(dictionary)来计算某个单词出现的次数。具体步骤如下:
1. 定义一个空字典,用于记录单词出现的次数。
2. 输入一段字符串,使用`split()`函数将其分割成单词列表。
3. 遍历单词列表,对于每个单词,如果它已经在字典中出现过,则将其对应的值加1,否则将其添加到字典中,并将其对应的值初始化为1。
4. 输出目标单词在字典中的值,即为其出现的次数。
下面是示例代码:
```python
word_count = {}
input_str = input("请输入一段文字:")
word_list = input_str.split() # 使用split()函数将输入的字符串分割成单词列表
for word in word_list:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
target_word = input("请输入要查询的单词:")
if target_word in word_count:
print(target_word, "出现了", word_count[target_word], "次。")
else:
print(target_word, "没有出现过。")
```
在上述代码中,我们首先定义了一个空字典`word_count`,然后使用`input()`函数获取用户输入的一段字符串,并使用`split()`函数将其分割成单词列表。接下来,我们对单词列表进行遍历,如果单词已经在字典中出现过,则将其对应的值加1,否则将其添加到字典中,并将其对应的值初始化为1。最后,我们输入要查询的单词,并判断其是否在字典中出现过,如果出现过,则输出其出现的次数,否则输出其没有出现过。
阅读全文
相关推荐
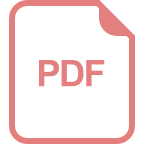
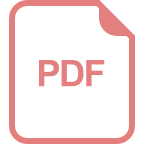
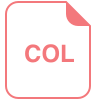
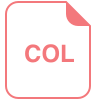
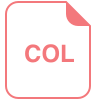
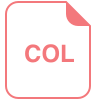
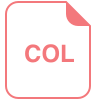
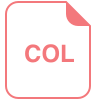
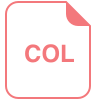




