python、已知二输入,一输出的数据用神经网络拟合曲面算法代码
时间: 2023-08-31 18:29:09 浏览: 77
### 回答1:
以下是一个简单的 Python 代码,用于使用神经网络拟合曲面:
```python
import numpy as np
import tensorflow as tf
from tensorflow import keras
# 生成训练数据
x1 = np.random.rand(100)
x2 = np.random.rand(100)
y = np.sin(x1) + np.cos(x2)
# 构建神经网络模型
model = keras.Sequential([
keras.layers.Dense(64, activation='relu', input_shape=(2,)),
keras.layers.Dense(64, activation='relu'),
keras.layers.Dense(1)
])
# 编译模型
model.compile(optimizer='adam', loss='mse')
# 训练模型
model.fit([x1, x2], y, epochs=100)
# 使用模型进行预测
x1_test = np.random.rand(10)
x2_test = np.random.rand(10)
y_pred = model.predict([x1_test, x2_test])
print(y_pred)
```
这个代码使用了一个具有两个输入和一个输出的神经网络模型,用于拟合一个二维曲面。训练数据是随机生成的,输出是输入的正弦和余弦之和。模型使用了两个隐藏层,每个隐藏层有 64 个神经元。模型使用了均方误差作为损失函数,使用 Adam 优化器进行训练。最后,模型使用随机生成的测试数据进行预测,并输出预测结果。
### 回答2:
在Python中,我们可以使用一种名为PyTorch的深度学习框架来实现神经网络拟合曲面的算法代码。
首先,我们需要导入必要的库和模块:
import torch
import torch.nn as nn
import torch.optim as optim
接下来,我们需要定义一个包含输入层、隐藏层和输出层的神经网络模型:
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.fc1 = nn.Linear(2, 16) # 输入层到隐藏层
self.fc2 = nn.Linear(16, 1) # 隐藏层到输出层
def forward(self, x):
x = torch.relu(self.fc1(x)) # 使用ReLU作为激活函数
x = self.fc2(x)
return x
然后,我们可以定义训练函数来训练我们的神经网络模型:
def train(model, optimizer, inputs, targets, num_epochs):
for epoch in range(num_epochs):
# 前向传播
outputs = model(inputs)
loss = criterion(outputs, targets)
# 反向传播及优化
optimizer.zero_grad()
loss.backward()
optimizer.step()
if (epoch+1) % 10 == 0:
print('Epoch [{}/{}], Loss: {:.4f}'.format(epoch+1, num_epochs, loss.item()))
接着,我们可以准备数据并创建模型实例:
# 准备数据
inputs = torch.tensor([[0, 0], [0, 1], [1, 0], [1, 1]], dtype=torch.float32)
targets = torch.tensor([[0], [1], [1], [0]], dtype=torch.float32)
# 创建模型实例
model = Net()
最后,我们需要定义损失函数和优化器,并调用训练函数开始训练:
# 定义损失函数和优化器
criterion = nn.MSELoss()
optimizer = optim.SGD(model.parameters(), lr=0.01)
# 开始训练
num_epochs = 100
train(model, optimizer, inputs, targets, num_epochs)
这样,通过多次迭代训练,我们的神经网络模型将逐渐拟合输入数据的曲面,并输出预测结果。在训练过程中,我们可以观察损失函数的变化情况来评估模型的训练效果。
### 回答3:
要使用神经网络拟合二输入一输出的数据曲面,可以使用Python中的一些机器学习库,例如TensorFlow或PyTorch来实现。以下是一个基本的使用TensorFlow来构建并训练神经网络的代码示例:
首先,我们导入所需的库:
```python
import tensorflow as tf
import numpy as np
```
然后,定义输入和输出的数据:
```python
# 定义输入数据
inputs = np.array([[1, 2],
[3, 4],
[5, 6],
...
])
# 定义输出数据
outputs = np.array([10, 20, 30, ...])
```
接下来,我们定义神经网络的结构和训练参数:
```python
# 定义神经网络结构
model = tf.keras.Sequential([
tf.keras.layers.Dense(64, activation='relu', input_shape=(2,)),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(1)
])
# 定义训练参数
model.compile(optimizer='adam',
loss='mean_squared_error',
metrics=['mse'])
```
然后,我们进行数据拆分,将数据分为训练集和测试集:
```python
# 数据拆分
train_inputs = inputs[:200]
train_outputs = outputs[:200]
test_inputs = inputs[200:]
test_outputs = outputs[200:]
```
接下来,我们使用拆分后的训练数据来训练神经网络:
```python
# 训练神经网络
model.fit(train_inputs, train_outputs, epochs=100, batch_size=32)
```
最后,我们使用测试数据评估神经网络性能:
```python
# 评估神经网络性能
loss, mse = model.evaluate(test_inputs, test_outputs)
print(f"Loss: {loss}, Mean Squared Error: {mse}")
```
以上是一个基本的使用TensorFlow来构建并训练神经网络进行数据拟合的示例代码。根据实际情况,你可能需要对模型结构进行调整、调整训练参数或进行其他的优化来得到更好的结果。
相关推荐
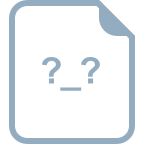
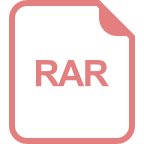














