mvc登录实例mysql_MVC(JSP+JavaBean+Servlet)入口实例:用户登录验证,要求有User、UserDao、UserController、login.jsp
时间: 2024-01-14 11:04:17 浏览: 25
好的,下面是一个简单的MVC登录实例,包含了User、UserDao、UserController和login.jsp。
首先是User类,用于表示用户信息:
```java
public class User {
private String username;
private String password;
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
}
```
接下来是UserDao类,用于与数据库交互:
```java
public class UserDao {
public User getUser(String username, String password) throws SQLException {
Connection conn = null;
PreparedStatement stmt = null;
ResultSet rs = null;
try {
conn = DBUtil.getConnection();
String sql = "select * from user where username=? and password=?";
stmt = conn.prepareStatement(sql);
stmt.setString(1, username);
stmt.setString(2, password);
rs = stmt.executeQuery();
if (rs.next()) {
User user = new User();
user.setUsername(rs.getString("username"));
user.setPassword(rs.getString("password"));
return user;
}
return null;
} finally {
DBUtil.close(rs, stmt, conn);
}
}
}
```
UserController类是控制器,负责处理用户请求和返回结果:
```java
public class UserController extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String username = request.getParameter("username");
String password = request.getParameter("password");
UserDao userDao = new UserDao();
User user = null;
try {
user = userDao.getUser(username, password);
} catch (SQLException e) {
e.printStackTrace();
}
if (user != null) {
request.getSession().setAttribute("user", user);
response.sendRedirect("index.jsp");
} else {
request.setAttribute("error", "用户名或密码错误");
request.getRequestDispatcher("login.jsp").forward(request, response);
}
}
}
```
最后是login.jsp页面,用于显示登录表单和错误信息:
```html
<!DOCTYPE html>
<html>
<head>
<title>登录</title>
</head>
<body>
<h1>登录</h1>
<form method="post" action="login">
<p>
<label>用户名:</label>
<input type="text" name="username">
</p>
<p>
<label>密码:</label>
<input type="password" name="password">
</p>
<p>
<input type="submit" value="登录">
</p>
</form>
<p style="color:red">${error}</p>
</body>
</html>
```
以上就是一个简单的MVC登录实例,其中使用了JSP、JavaBean和Servlet实现了用户登录验证功能。
相关推荐
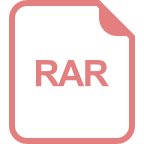
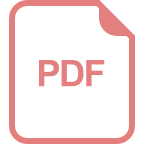














