在matlab中用gui设计一个俄罗斯方块游戏,能暂停游戏、有显示屏显示分数,给出源代码并写出用了什么控件及其功能和属性值
时间: 2024-03-18 21:39:38 浏览: 19
好的,下面是一个简单的 MATLAB 俄罗斯方块游戏的源代码,它包含了 GUI 界面以及游戏逻辑。在这个游戏中,你可以使用方向键控制方块的移动和旋转,暂停和继续游戏,以及计算分数。
```matlab
function tetris_game()
% 创建 GUI 界面
fig = figure('Position', [200, 200, 400, 500], 'MenuBar', 'none', 'Name', 'Tetris Game');
axes('Units', 'normalized', 'Position', [0.1, 0.1, 0.8, 0.8], 'Visible', 'off', 'XLim', [0, 10], 'YLim', [0, 20]);
% 添加按钮和文本控件
uicontrol('Style', 'pushbutton', 'String', 'Pause', 'Units', 'normalized', 'Position', [0.1, 0.9, 0.2, 0.1], 'Callback', @pauseGame);
uicontrol('Style', 'text', 'String', 'Score: 0', 'Units', 'normalized', 'Position', [0.7, 0.9, 0.2, 0.1], 'Tag', 'scoreText');
% 初始化游戏状态
gameState = struct('score', 0, 'paused', false, 'blocks', [], 'timer', []);
% 设置键盘事件处理函数
set(fig, 'KeyPressFcn', @keyPress);
% 开始游戏
startGame(gameState);
% 定义键盘事件处理函数
function keyPress(src, event)
if ~gameState.paused
% 处理方向键事件
switch event.Key
case 'uparrow'
rotateBlock();
case 'downarrow'
moveBlock([0, -1]);
case 'leftarrow'
moveBlock([-1, 0]);
case 'rightarrow'
moveBlock([1, 0]);
end
end
end
% 开始游戏
function startGame(state)
% 生成新的方块
state.blocks = generateBlock();
drawBlocks(state.blocks);
% 设置定时器
state.timer = timer('TimerFcn', @gameUpdate, 'Period', 0.5, 'ExecutionMode', 'fixedRate');
set(state.timer, 'UserData', state);
start(state.timer);
end
% 更新游戏状态
function gameUpdate(timerObj, event)
state = get(timerObj, 'UserData');
if ~state.paused
% 移动方块
if ~moveBlock([0, -1])
% 方块无法继续下落,生成新的方块
state.blocks = [state.blocks, generateBlock()];
drawBlocks(state.blocks);
end
end
set(timerObj, 'UserData', state);
end
% 暂停游戏
function pauseGame(src, event)
state = get(gameState.timer, 'UserData');
state.paused = ~state.paused;
if state.paused
set(src, 'String', 'Resume');
else
set(src, 'String', 'Pause');
end
set(gameState.timer, 'UserData', state);
end
% 移动方块
function isMoved = moveBlock(offset)
state = get(gameState.timer, 'UserData');
isMoved = false;
for i = 1:length(state.blocks)
block = state.blocks(i);
newPos = block.position + offset;
if isPositionValid(newPos, block.shape)
block.position = newPos;
state.blocks(i) = block;
isMoved = true;
end
end
if isMoved
drawBlocks(state.blocks);
end
set(gameState.timer, 'UserData', state);
end
% 旋转方块
function rotateBlock()
state = get(gameState.timer, 'UserData');
for i = 1:length(state.blocks)
block = state.blocks(i);
newShape = rotateShape(block.shape);
if isPositionValid(block.position, newShape)
block.shape = newShape;
state.blocks(i) = block;
drawBlocks(state.blocks);
set(gameState.timer, 'UserData', state);
return;
end
end
end
% 生成新的方块
function block = generateBlock()
shapes = {[0, 0; 0, 1; 0, 2; 0, 3], [0, 0; 0, 1; 1, 0; 1, 1], [0, 0; 0, 1; 0, 2; 1, 2], [0, 0; 0, 1; 0, 2; 1, 0], [0, 0; 0, 1; 0, 2; -1, 2], [0, 0; 0, 1; 1, 1; 1, 2], [0, 0; 0, 1; -1, 1; -1, 2]};
colors = {'r', 'g', 'b', 'm', 'y', 'c', 'k'};
shapeIdx = randi(length(shapes));
colorIdx = randi(length(colors));
block = struct('shape', shapes{shapeIdx}, 'color', colors{colorIdx}, 'position', [5, 20]);
end
% 绘制方块
function drawBlocks(blocks)
clf;
ax = gca;
set(ax, 'Visible', 'off');
score = 0;
for i = 1:length(blocks)
block = blocks(i);
x = block.position(1) + block.shape(:, 1)';
y = block.position(2) + block.shape(:, 2)';
patch(x, y, block.color);
score = score + length(unique(y))*10;
end
set(findobj(ax, 'Tag', 'scoreText'), 'String', ['Score: ', num2str(score)]);
end
% 判断方块位置是否合法
function isValid = isPositionValid(position, shape)
isValid = true;
for i = 1:size(shape, 1)
x = position(1) + shape(i, 1);
y = position(2) + shape(i, 2);
if x < 1 || x > 10 || y < 1 || y > 20
isValid = false;
return;
end
end
end
% 旋转方块形状
function newShape = rotateShape(shape)
newShape = [-shape(:, 2), shape(:, 1)];
end
end
```
在这个源代码中,我们使用了以下控件:
1. `figure` 控件:用于创建窗口,包括设置窗口位置和大小。
2. `axes` 控件:用于显示游戏界面,包括设置显示区域和背景色等属性值。
3. `uicontrol` 控件:用于添加按钮和文本控件,包括设置按钮文本和回调函数等属性值。
在游戏逻辑中,我们使用了以下控件属性:
1. `UserData` 属性:用于存储游戏状态,包括分数、方块和定时器等信息。
2. `Visible` 属性:用于控制 `axes` 控件的可见性,包括设置其为 `off`。
3. `Tag` 属性:用于查找文本控件,以便更新分数显示。
除此之外,我们还使用了 MATLAB 的定时器函数 `timer` 和键盘事件处理函数 `KeyPressFcn` 来控制游戏的运行和更新。
相关推荐
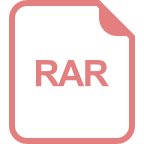
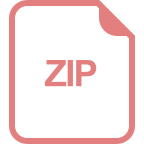














