qt opencv线程存图加上线程锁
时间: 2023-05-16 15:05:06 浏览: 125
可以使用 Qt 的 QThread 类来创建线程,使用 OpenCV 的 VideoCapture 类来获取视频帧,然后使用 OpenCV 的 imwrite 函数将图像保存到磁盘上。为了避免多个线程同时访问同一个图像,可以使用互斥锁来保护共享资源。以下是示例代码:
```cpp
#include <QtCore>
#include <opencv2/opencv.hpp>
class WorkerThread : public QThread
{
Q_OBJECT
public:
WorkerThread(QObject *parent = nullptr)
: QThread(parent)
{
}
void run() override
{
cv::VideoCapture cap(0);
if (!cap.isOpened()) {
emit error("Failed to open camera");
return;
}
cv::Mat frame;
for (;;) {
cap >> frame;
if (frame.empty()) {
emit error("Failed to capture frame");
break;
}
// Lock the mutex before accessing the shared resource
mutex.lock();
// Save the image to disk
cv::imwrite("frame.jpg", frame);
// Unlock the mutex after accessing the shared resource
mutex.unlock();
}
}
signals:
void error(const QString &msg);
private:
QMutex mutex;
};
int main(int argc, char *argv[])
{
QCoreApplication app(argc, argv);
WorkerThread thread;
QObject::connect(&thread, &WorkerThread::error, &app, [](const QString &msg) {
qDebug() << "Error:" << msg;
});
thread.start();
return app.exec();
}
#include "main.moc"
```
这个程序使用了一个 WorkerThread 类来处理视频帧的获取和保存。在 run 函数中,它首先创建了一个 VideoCapture 对象来获取摄像头的视频帧。然后,它循环读取每一帧,并使用 imwrite 函数将图像保存到磁盘上。在访问共享资源之前,它使用了一个互斥锁来保护它。这样可以确保多个线程不会同时访问同一个图像。
阅读全文
相关推荐
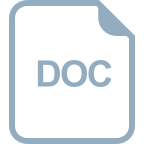
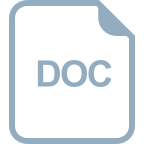
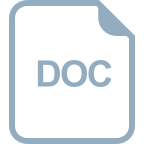

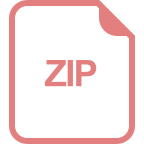
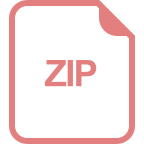
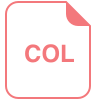
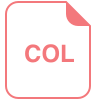





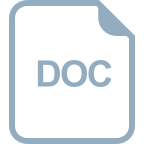
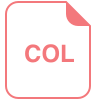
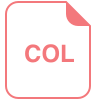
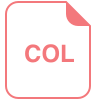
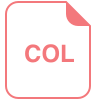
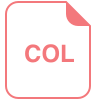