Gui界面mnist手写数字识别
时间: 2023-09-07 20:14:42 浏览: 116
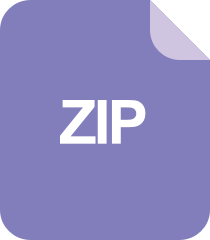
基于MNIST数据集卷积神经网络实现手写数字识别(GUI)

要实现GUI界面的MNIST手写数字识别,您可以使用Python的Tkinter模块来创建GUI界面,并使用Python的Keras库来构建和训练数字分类模型。以下是一个简单的实现步骤:
1. 导入所需的模块和库:
```
from tkinter import *
from keras.models import load_model
from PIL import Image, ImageDraw
import numpy as np
```
2. 创建GUI界面:
```
root = Tk()
root.title("MNIST手写数字识别")
root.geometry('280x280')
canvas = Canvas(root, width=280, height=280, bg='white')
canvas.pack()
image = Image.new("L", (280, 280), 0)
draw = ImageDraw.Draw(image)
```
3. 加载预训练的Keras模型:
```
model = load_model('mnist_model.h5')
```
4. 定义画布上的鼠标事件:
```
def paint(event):
x1, y1 = (event.x - 10), (event.y - 10)
x2, y2 = (event.x + 10), (event.y + 10)
canvas.create_oval(x1, y1, x2, y2, fill='black', width=10)
draw.line([x1, y1, x2, y2], fill='white', width=10)
canvas.bind('<B1-Motion>', paint)
```
5. 定义识别手写数字的函数:
```
def recognize():
digit = image.resize((28, 28))
digit = np.array(digit)
digit = digit.reshape(1, 28, 28, 1)
digit = digit.astype('float32')
digit /= 255.0
result = model.predict(digit)
result = np.argmax(result)
label_result.config(text="识别结果:" + str(result))
```
6. 添加按钮和标签:
```
btn_recognize = Button(root, text="识别", command=recognize)
btn_recognize.pack(side=BOTTOM)
label_result = Label(root, text="")
label_result.pack(side=BOTTOM)
```
7. 运行GUI界面:
```
root.mainloop()
```
这样,您就可以在GUI界面上手写数字,点击“识别”按钮,识别结果将显示在标签上。
阅读全文
相关推荐
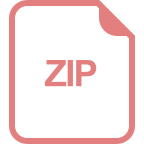
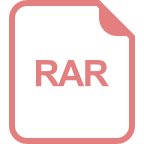















