struct Vertex { float x, y, z; }; std::ostream& operator<<(std::ostream& stream, const Vertex& vertex) { stream << vertex.x << "," << vertex.y << "," << vertex.z; return stream; } int main() { std::vector<Vertex>vertices; vertices.push_back({ 1,2,3 }); vertices.push_back({ 4,5,6 }); for (Vertex v : vertices) { cout << v << endl; } cin.get(); }里面的for循环参数该如何理解,是怎么工作的
时间: 2024-02-15 13:04:35 浏览: 31
这个 for 循环使用了范围 for 循环语法,也称为 for-each 循环。这种语法可以用来遍历一组值,例如一个容器中的所有元素。在这个例子中,for 循环遍历了 `vertices` 容器中的每个元素,每个元素都是一个 `Vertex` 类型的对象。
在循环体中,使用了一个输出运算符重载函数,它接收一个输出流和一个 `const Vertex&` 类型的对象引用,将 `Vertex` 对象的 `x`、`y`、`z` 成员变量按照一定的格式输出到输出流中。在循环中,每个 `Vertex` 对象都会被输出到控制台上,每个对象的输出都会换行,因此控制台上会输出两行内容,分别是:
```
1,2,3
4,5,6
```
这个例子中的输出运算符重载函数是为了方便输出 `Vertex` 对象的数据,它不会影响循环的工作方式。循环的参数是 `Vertex v`,这意味着在每次循环迭代中,变量 `v` 都会被初始化为一个容器中的 `Vertex` 对象,然后在循环体中使用。
相关问题
帮我挑错误:#include <iostream> #include <any> #include <data_structures> using namespace std; class Any { template <class T> friend std::ostream& operator << (std::ostream& out, const Any& a); struct S { virtual ~S() = default; } *s; template <class T> struct Data : S { Data(T dt) : data(dt) {} T data; }; public: template <class T> Any(T data) : s(new Data<T>(data)) {} ~Any() { delete s; } template <class T> void operator = (T data) { delete s; s = new Data<T>(data); } template <class T> T _Cast() { return dynamic_cast<Data<T>*>(s) -> data; } }; template <class T> std::ostream& operator << (std::ostream& out, const Any& a) { out << a._Cast<T>(); return out; } int main() { Any a = 12; cout << a << endl; a = 'a'; cout << a << endl; return 0; }
这段代码有几个错误:
1. 在 `#include` 中引入了一个名为 `data_structures` 的头文件,但是 C++ 标准库中没有这个头文件。
2. 在类 `Any` 中定义了一个名为 `Any` 的构造函数,这会引起命名冲突。
3. `Any` 类中的 `operator=` 函数没有处理自我赋值的情况,在执行 `delete s;` 后,如果 `s` 指向的是自身,则会导致未定义行为。
4. `_Cast` 函数没有处理无效的类型转换,如果尝试将 `Any` 对象转换为不匹配的类型,可能会导致运行时错误。
下面是修正后的代码:
```cpp
#include <iostream>
using namespace std;
class Any
{
template <class T>
friend std::ostream& operator << (std::ostream& out, const Any& a);
struct S { virtual ~S() = default; };
template <class T>
struct Data : S
{
Data(T dt) : data(dt) {}
T data;
};
public:
template <class T>
Any(T data) : s(new Data<T>(data)) {}
~Any() { delete s; }
template <class T>
Any& operator = (const T& data)
{
if (this == &data) {
return *this;
}
delete s;
s = new Data<T>(data);
return *this;
}
template <class T>
T _Cast() const
{
Data<T>* castedData = dynamic_cast<Data<T>*>(s);
if (castedData) {
return castedData->data;
}
throw std::bad_cast();
}
private:
S* s;
};
template <class T>
std::ostream& operator << (std::ostream& out, const Any& a)
{
out << a._Cast<T>();
return out;
}
int main()
{
Any a = 12;
cout << a << endl;
a = 'a';
cout << a << endl;
return 0;
}
```
修正后的代码中删除了无用的头文件 `data_structures`,修复了命名冲突问题,添加了自我赋值检查,处理了无效的类型转换,并将 `_Cast` 函数标记为 `const`,以便在不修改对象的情况下进行类型转换。
no type named 'type' in 'struct std::enable_if<false, std::basic_ostream<char>&>'
这个错误通常是因为模板参数导致的。在使用 `enable_if` 时,如果条件为 false,那么模板参数就不存在,因此编译器会报出这个错误。你可能需要检查一下使用 `enable_if` 的地方,看看是否有以下几个问题:
1. 条件表达式是否正确。`enable_if` 的第一个模板参数是一个条件表达式,如果这个表达式的值为 false,那么 `enable_if` 就无法实例化,从而导致上述错误。
2. `enable_if` 是否在正确的位置。`enable_if` 应该出现在模板参数列表中,用于控制这个模板参数是否被实例化。如果 `enable_if` 出现在错误的位置,也会导致上述错误。
3. 是否正确地使用了 `typename` 关键字。在模板中使用嵌套类型时,需要使用 `typename` 关键字来告诉编译器这是一个类型而不是一个成员变量。如果忘记使用 `typename` 关键字,也会导致上述错误。
希望这些提示能对你有所帮助。
相关推荐
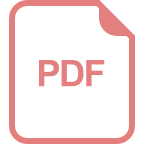
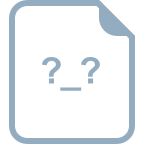
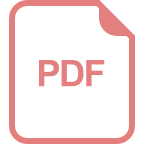












