(a) 总结 GCC 和 GDB 的用法 (b) 按照讲义上的例子,自己生成静态库和动态库。 (c) 预习讲义Linux_4_文件, 并写程序完成下面题目 (每道小题对应一个程序)。 1) 写程序创建 ~/tmp 目录。 2)写程序删除 ~/tmp 2) 写程序实现类似 ls 的效果 (只显示文件名)。 // list.c int main(int argc, char* argv[]) { // ./list dir }
时间: 2024-02-15 20:04:26 浏览: 18
(a) GCC是GNU编译器套件中的一个编译器,可以将C、C ++、Objective-C、Fortran、Ada和其他语言的源代码编译成可执行文件。GDB是GNU项目中的调试器,可以用于调试各种编程语言编写的程序。
(b) 生成静态库:使用ar工具将多个目标文件打包成一个归档文件,命令为:ar rcs libtest.a test1.o test2.o。生成动态库:使用gcc编译时加上-shared选项,命令为:gcc -shared -o libtest.so test1.o test2.o。
(c)
1) 创建~/tmp目录的程序:
```c
#include <stdio.h>
#include <sys/stat.h>
#include <sys/types.h>
int main() {
char* path = "/home/user/tmp";
int status = mkdir(path, S_IRWXU | S_IRWXG | S_IROTH | S_IXOTH);
if (status != 0) {
printf("Error creating directory\n");
return 1;
}
printf("Directory created successfully\n");
return 0;
}
```
2) 删除~/tmp目录的程序:
```c
#include <stdio.h>
#include <unistd.h>
int main() {
char* path = "/home/user/tmp";
int status = rmdir(path);
if (status != 0) {
printf("Error deleting directory\n");
return 1;
}
printf("Directory deleted successfully\n");
return 0;
}
```
3) 实现类似ls的效果的程序:
```c
#include <dirent.h>
#include <stdio.h>
#include <stdlib.h>
int main(int argc, char* argv[]) {
char* path = ".";
if (argc > 1) {
path = argv[1];
}
DIR* dir = opendir(path);
if (dir == NULL) {
printf("Error opening directory\n");
return 1;
}
struct dirent* entry;
while ((entry = readdir(dir)) != NULL) {
printf("%s\n", entry->d_name);
}
closedir(dir);
return 0;
}
```
相关推荐
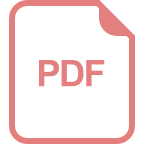
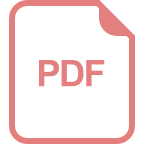
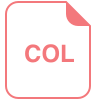
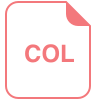
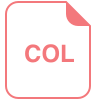
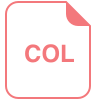
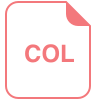









