winform 使用异步方式编写http协议服务端
时间: 2023-07-30 13:07:32 浏览: 191
在WinForms中,可以使用异步方式来编写HTTP协议服务端,以便不会阻塞UI线程。
以下是一个简单的例子,演示如何使用异步方式编写HTTP协议服务端:
```csharp
public partial class Form1 : Form
{
private HttpListener httpListener;
public Form1()
{
InitializeComponent();
}
private async void btnStart_Click(object sender, EventArgs e)
{
try
{
httpListener = new HttpListener();
httpListener.Prefixes.Add("http://localhost:8080/");
httpListener.Start();
txtStatus.Text = "HTTP server is running on port 8080.";
while (true)
{
HttpListenerContext context = await httpListener.GetContextAsync();
Task.Run(() => ProcessRequest(context));
}
}
catch (Exception ex)
{
txtStatus.Text = "Error: " + ex.Message;
}
}
private void ProcessRequest(HttpListenerContext context)
{
try
{
string responseString = "<html><body><h1>Hello, World!</h1></body></html>";
byte[] buffer = Encoding.UTF8.GetBytes(responseString);
context.Response.ContentLength64 = buffer.Length;
context.Response.OutputStream.Write(buffer, 0, buffer.Length);
context.Response.OutputStream.Close();
}
catch (Exception ex)
{
// Handle exception
}
}
private void btnStop_Click(object sender, EventArgs e)
{
httpListener.Stop();
txtStatus.Text = "HTTP server stopped.";
}
}
```
在上面的例子中,我们使用了HttpListener类来创建一个HTTP协议服务端,并监听端口8080。在启动按钮的Click事件中,我们使用async关键字来异步处理HTTP请求,并使用Task.Run方法来处理每个请求。在处理HTTP请求的方法中,我们简单地返回一个包含“Hello, World!”文本的HTML响应。在停止按钮的Click事件中,我们停止HttpListener并更新状态文本框。
阅读全文
相关推荐











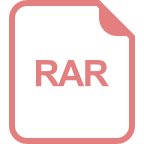




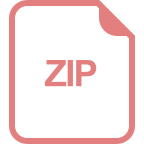

