树莓派颜色识别代码
时间: 2023-08-03 19:07:27 浏览: 171
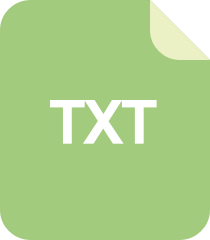
树莓派上传识别颜色代码
以下是一个基于Python和OpenCV的树莓派颜色识别代码示例,可以进行颜色检测并在三色 LED 灯上显示相应的颜色:
```python
import cv2
import numpy as np
import RPi.GPIO as GPIO
import time
# 设置三色 LED 灯的引脚号
red_pin = 17
green_pin = 27
blue_pin = 22
# 初始化 GPIO 引脚
GPIO.setmode(GPIO.BCM)
GPIO.setup(red_pin, GPIO.OUT)
GPIO.setup(green_pin, GPIO.OUT)
GPIO.setup(blue_pin, GPIO.OUT)
# 定义颜色范围(在 HSV 颜色空间中)
color_range = {
'red': [(0, 100, 100), (10, 255, 255)],
'green': [(36, 25, 25), (86, 255, 255)],
'blue': [(100, 50, 50), (130, 255, 255)]
}
# 定义函数:根据颜色范围检测图像中的颜色
def detect_color(img, color):
hsv = cv2.cvtColor(img, cv2.COLOR_BGR2HSV)
lower = np.array(color_range[color][0])
upper = np.array(color_range[color][1])
mask = cv2.inRange(hsv, lower, upper)
return mask
# 初始化摄像头
cap = cv2.VideoCapture(0)
cap.set(3, 640)
cap.set(4, 480)
# 开始循环
while True:
# 读取摄像头拍摄的画面
ret, frame = cap.read()
# 根据颜色范围检测红色、绿色和蓝色
red_mask = detect_color(frame, 'red')
green_mask = detect_color(frame, 'green')
blue_mask = detect_color(frame, 'blue')
# 计算红色、绿色和蓝色的像素面积
red_pixels = cv2.countNonZero(red_mask)
green_pixels = cv2.countNonZero(green_mask)
blue_pixels = cv2.countNonZero(blue_mask)
# 根据像素面积判断检测到的颜色
if red_pixels > green_pixels and red_pixels > blue_pixels:
GPIO.output(red_pin, True)
GPIO.output(green_pin, False)
GPIO.output(blue_pin, False)
elif green_pixels > red_pixels and green_pixels > blue_pixels:
GPIO.output(red_pin, False)
GPIO.output(green_pin, True)
GPIO.output(blue_pin, False)
elif blue_pixels > red_pixels and blue_pixels > green_pixels:
GPIO.output(red_pin, False)
GPIO.output(green_pin, False)
GPIO.output(blue_pin, True)
# 显示检测结果
cv2.imshow('frame', frame)
# 等待按下 ESC 键退出
k = cv2.waitKey(5) & 0xFF
if k == 27:
break
# 释放摄像头并清空窗口
cap.release()
cv2.destroyAllWindows()
# 关闭 GPIO 引脚
GPIO.cleanup()
```
在运行程序之前,需要先将三色 LED 灯连接到树莓派的GPIO引脚上,并根据程序中定义的引脚号进行连接。同时,需要安装OpenCV库和RPi.GPIO库。运行程序后,将不同颜色的物体放在摄像头前,观察程序是否能够正确识别颜色并控制三色 LED 灯显示对应的颜色。
阅读全文
相关推荐
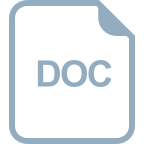
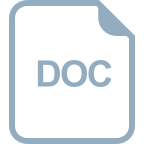
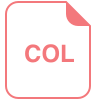
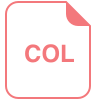
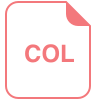
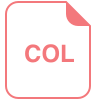
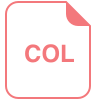
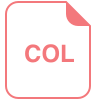







