element-ui Vue-socket.io
时间: 2024-08-12 17:05:55 浏览: 47
Element UI是一个基于Vue.js的开源UI组件库,它提供了一系列美观、易用并且高度交互的现成组件,帮助开发者快速构建响应式的Web应用。Element UI的设计风格简洁现代,覆盖了从基础的布局到复杂的表单和数据展示等各个方面。
Vue-socket.io则是Vue.js生态下的一个插件,用于在Vue应用中集成Socket.IO,这是一个实时Web应用程序库,使得前端能够与服务器进行全双工通信,实现实时消息推送、在线用户管理等功能。通过Vue-socket.io,开发者可以轻松地处理WebSocket连接,并将实时通信能力融入到Vue应用中,创建需要即时反馈的应用,如聊天室、游戏等。
相关问题
vue.js+element ui+node.js+socket.io+mysql
Vue.js 是一种现代的JavaScript框架,用于构建向响应式Web应用程序。它的核心思想是将大型应用程序分割成更小的,可重用的组件。
Element UI 是Vue.js的一个组件框架,提供了许多可轻松集成到Vue.js应用程序中,并对HTML和CSS进行了巧妙组合的组件。
Node.js 是JavaScript运行时环境,使得开发人员可以在服务器端使用JavaScript编写代码。它是一种轻量级的技术堆栈和可扩展Web服务器, 由于它非阻塞的特性,具有高并发性能。
Socket.io 是WebSocket库,它使得客户端和服务器之间可以实时交互数据,而不必等待HTTP的请求和响应的时间延迟时间,从而实现了实时的双向通信。
MySQL 是一个关系型数据库管理系统,是全球最流行的开源数据存储,用于存储和管理数据。
这些技术的组合可以用于构建高性能,实时响应,具有良好用户体验的Web应用程序。 例如,可以使用Vue.js和Element UI开发具有漂亮的前端设计的应用程序,使用不阻塞I/O的特性和事件驱动的架构,Node.js可以实现高效的后端服务。而MySQL数据库可以处理数据存储和检索。通过与Socket.io集成,可以实现实时交互和数据推送,增强应用程序的实时性。因此可以看到,Vue.js, Element UI, Node.js, Socket.io和MySQL 这些技术的组合,是构建响应式,高性能,可扩展Web应用程序之所需技术栈。
用vue 和 element 生成一份视频会议UI界面
好的,下面是一个简单的视频会议UI界面的示例代码,使用Vue和Element UI库实现:
```html
<template>
<div class="video-meeting">
<el-row>
<el-col :span="16">
<div class="video-container">
<video ref="localVideo" autoplay="autoplay"></video>
<video ref="remoteVideo" autoplay="autoplay"></video>
</div>
<div class="btn-group">
<el-button
type="primary"
icon="el-icon-video-camera"
@click="toggleCamera"
:disabled="!peerConnection"
>
{{ isCameraOn ? '关闭摄像头' : '开启摄像头' }}
</el-button>
<el-button
type="primary"
icon="el-icon-microphone"
@click="toggleMic"
:disabled="!peerConnection"
>
{{ isMicOn ? '关闭麦克风' : '开启麦克风' }}
</el-button>
<el-button
type="primary"
icon="el-icon-phone"
@click="hangup"
:disabled="!peerConnection"
>
挂断
</el-button>
</div>
</el-col>
<el-col :span="8">
<el-form :model="form" label-position="top" label-width="80px">
<el-form-item label="会议号">
<el-input v-model="form.meetingId"></el-input>
</el-form-item>
<el-form-item label="用户名">
<el-input v-model="form.username"></el-input>
</el-form-item>
<el-form-item>
<el-button type="primary" @click="joinMeeting">加入会议</el-button>
</el-form-item>
</el-form>
<ul v-if="userList.length" class="user-list">
<li v-for="(user, index) in userList" :key="index">{{ user }}</li>
</ul>
</el-col>
</el-row>
</div>
</template>
<script>
import { mapState } from 'vuex'
export default {
name: 'VideoMeeting',
data() {
return {
form: {
meetingId: '',
username: ''
},
isCameraOn: true,
isMicOn: true,
peerConnection: null,
localStream: null,
remoteStream: null,
userList: []
}
},
computed: {
...mapState(['socket'])
},
created() {
navigator.mediaDevices.getUserMedia({
video: true,
audio: true
}).then(stream => {
this.$refs.localVideo.srcObject = stream
this.localStream = stream
}).catch(error => {
console.log(error)
})
},
methods: {
toggleCamera() {
this.isCameraOn = !this.isCameraOn
this.localStream.getVideoTracks()[0].enabled = this.isCameraOn
},
toggleMic() {
this.isMicOn = !this.isMicOn
this.localStream.getAudioTracks()[0].enabled = this.isMicOn
},
createPeerConnection() {
const pcConfig = {
iceServers: [
{
urls: 'stun:stun.l.google.com:19302'
}
]
}
this.peerConnection = new RTCPeerConnection(pcConfig)
this.peerConnection.onicecandidate = event => {
if (event.candidate) {
this.socket.emit('icecandidate', {
meetingId: this.form.meetingId,
username: this.form.username,
candidate: event.candidate
})
}
}
this.peerConnection.onaddstream = event => {
this.remoteStream = event.stream
this.$refs.remoteVideo.srcObject = this.remoteStream
}
this.peerConnection.addStream(this.localStream)
},
joinMeeting() {
this.createPeerConnection()
this.socket.emit('joinMeeting', {
meetingId: this.form.meetingId,
username: this.form.username
})
this.socket.on('userList', userList => {
this.userList = userList
})
this.socket.on('offer', async data => {
try {
await this.peerConnection.setRemoteDescription(new RTCSessionDescription(data.offer))
const answer = await this.peerConnection.createAnswer()
await this.peerConnection.setLocalDescription(answer)
this.socket.emit('answer', {
meetingId: this.form.meetingId,
username: this.form.username,
answer: answer
})
} catch (error) {
console.log(error)
}
})
this.socket.on('answer', async data => {
try {
await this.peerConnection.setRemoteDescription(new RTCSessionDescription(data.answer))
} catch (error) {
console.log(error)
}
})
this.socket.on('icecandidate', async data => {
try {
await this.peerConnection.addIceCandidate(new RTCIceCandidate(data.candidate))
} catch (error) {
console.log(error)
}
})
},
hangup() {
this.socket.emit('hangup', {
meetingId: this.form.meetingId,
username: this.form.username
})
this.peerConnection.close()
this.$router.push('/')
}
}
}
</script>
<style scoped>
.video-meeting {
margin: 20px;
.video-container {
display: flex;
justify-content: space-between;
align-items: center;
height: 500px;
video {
max-width: 48%;
max-height: 100%;
}
}
.btn-group {
display: flex;
justify-content: center;
margin-top: 20px;
button {
margin: 0 10px;
}
}
.user-list {
margin-top: 20px;
li {
margin-bottom: 10px;
}
}
}
</style>
```
其中使用了WebRTC技术实现视频通话,需要在调用getUserMedia方法前获取用户授权。同时,需要使用Socket.io库实现用户加入和退出会议、发送和接收offer、answer和icecandidate等操作。在代码中,使用了Vuex来管理Socket.io实例,需要在store中定义state和mutations。
此外,还使用了Element UI库提供的组件,如Row、Col、Form、Input和Button等,使界面更加美观和易用。
阅读全文
相关推荐
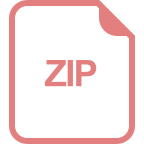
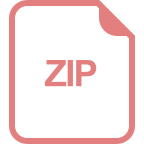
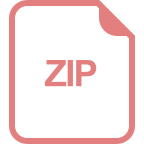
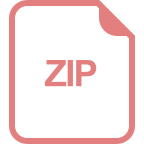
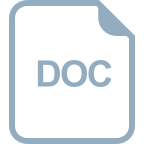
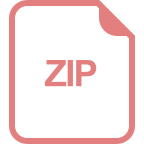
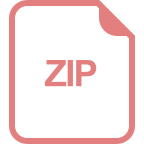
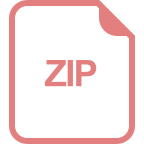
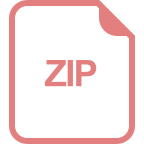
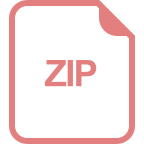
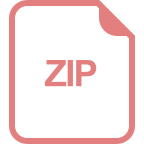
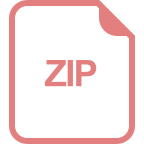
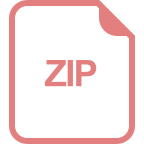
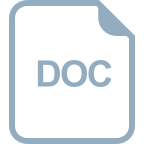
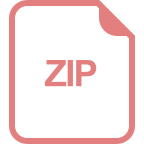