将总评成绩平均分以上的学生的等级确定为“均分以上”,同时将这些同学的对应信息存放在结构体数组h中,人数通过函数返回值得到.#include<studio.h>#include<string.h>
时间: 2023-12-28 11:06:27 浏览: 177
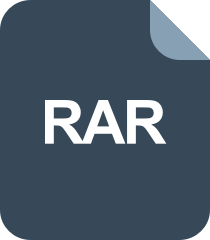
xueshengguanli.rar_学生_学生成绩
好的,这是一个关于学生成绩管理的问题,我可以帮你解答。
首先,我们需要定义一个结构体 `student`,用于存储每个学生的信息,包括姓名、学号、成绩等。
```C
struct student {
char name[20]; // 姓名
char id[10]; // 学号
float score; // 成绩
};
```
然后,我们需要读入所有学生的信息,并计算总平均分。
```C
int n; // 学生人数
scanf("%d", &n);
float sum = 0; // 所有学生的总分数
struct student h[100]; // 结构体数组,用于存放均分以上的学生信息
int cnt = 0; // 均分以上的学生人数
for (int i = 0; i < n; i++) {
scanf("%s %s %f", h[i].name, h[i].id, &h[i].score);
sum += h[i].score;
}
float avg = sum / n; // 所有学生的平均分数
```
接下来,我们遍历所有学生的成绩,将均分以上的学生信息存放到结构体数组 `h` 中,并统计人数。
```C
for (int i = 0; i < n; i++) {
if (h[i].score >= avg) {
strcpy(h[cnt].name, h[i].name);
strcpy(h[cnt].id, h[i].id);
h[cnt].score = h[i].score;
cnt++;
}
}
```
最后,我们返回均分以上的学生人数即可。
```C
return cnt;
```
完整代码如下:
```C
#include <stdio.h>
#include <string.h>
struct student {
char name[20]; // 姓名
char id[10]; // 学号
float score; // 成绩
};
int find_above_average(struct student h[], int n) {
float sum = 0; // 所有学生的总分数
int cnt = 0; // 均分以上的学生人数
for (int i = 0; i < n; i++) {
scanf("%s %s %f", h[i].name, h[i].id, &h[i].score);
sum += h[i].score;
}
float avg = sum / n; // 所有学生的平均分数
for (int i = 0; i < n; i++) {
if (h[i].score >= avg) {
strcpy(h[cnt].name, h[i].name);
strcpy(h[cnt].id, h[i].id);
h[cnt].score = h[i].score;
cnt++;
}
}
return cnt;
}
int main() {
struct student h[100];
int n = 5;
int cnt = find_above_average(h, n);
printf("均分以上的学生人数为:%d\n", cnt);
for (int i = 0; i < cnt; i++) {
printf("%s %s %.2f\n", h[i].name, h[i].id, h[i].score);
}
return 0;
}
```
阅读全文
相关推荐
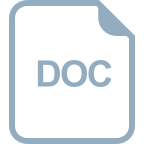
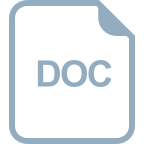









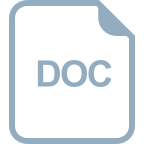
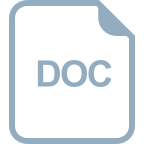
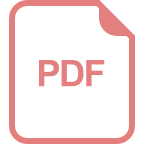
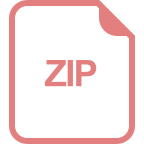
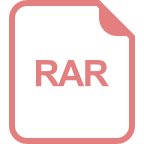
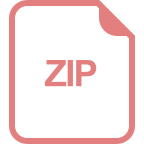
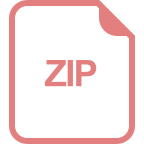